Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
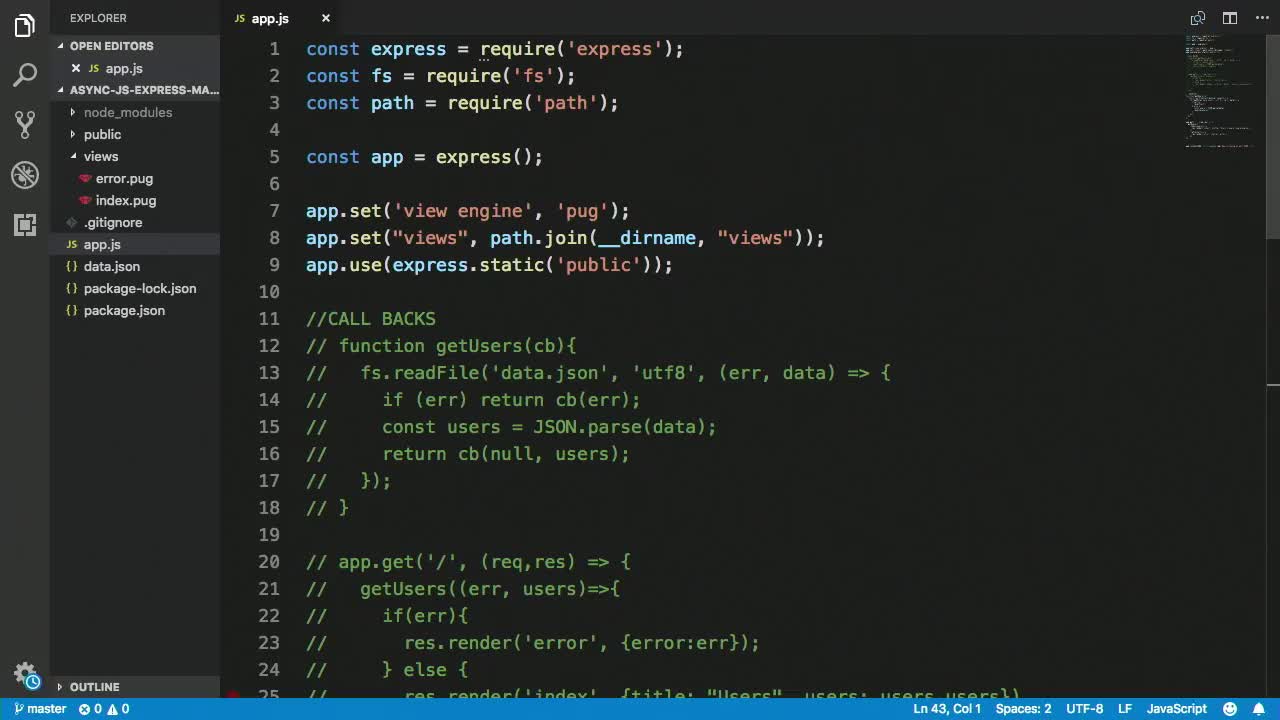
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
An example of using async/await syntax to handle asynchronous tasks in Express.
What two asynchronous operations might look like using async/await:
app.get('/:id', async (req, res) => {
try {
const user = await getUser(req.params.id);
const followers = await getFollowers(user);
res.render('profile', {title: "Profile Page", user: user, followers: followers});
} catch(err){
res.render('error', {error: err});
}
});
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
David Aguirre
8,170 Points1 Answer
-
Anthony Ogounchi
Full Stack JavaScript Techdegree Graduate 14,594 Points1 Answer
-
Luis Walderdorff
Full Stack JavaScript Techdegree Graduate 27,070 Points1 Answer
-
Vitaly Khe
7,160 Points2 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up