Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial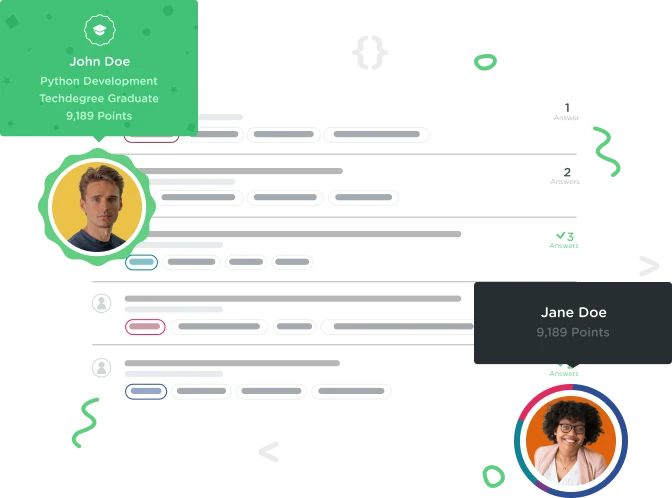
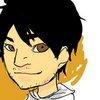
Ryo Yamamoto
9,165 PointsI'm getting the right answer if I run this code, but it's giving me an error "Didn't get the right number of teachers!"
I'm getting the right answer if I run this code, but it's giving me an error "Didn't get the right number of teachers!" Can anyone know what would be the reason?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
dictionary = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def num_teachers(dictionary):
for key in dictionary.keys():
num = len(key.split())
return num
3 Answers
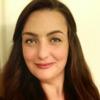
Jennifer Nordell
Treehouse TeacherHi there! You're getting the correct number, but for the wrong reasons. This will be a bit more obvious if you add a third teacher to your list. You'll see that it still returns 2. And there's a couple of things going on. First, you're splitting the key on all whitespace which means that "Andrew" and "Chalkley" now count as two separate teachers. This is where you got your count of 2. Immediate afterward you then return that number. Remember, a function ceases to execute the first time a return
statement is hit. So it only ever looks at the key "Andrew Chalkley" and then splits that key into 2 then returns that number.
In my opinion, there is a far simpler way to do this that doesn't require a loop at all. I chose to do it like this:
def num_teachers(dictionary):
return len(dictionary.keys());
Because the .keys()
method returns a list of all available keys as documented here, I chose to return the length of the list of those keys.
Hope this helps!
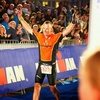
Steve Hunter
57,712 PointsHi there,
If you're going to iterate over the keys, start with a variable that's set to zero and increment it within the for
loop. Return that variable after the loop.
Steve.
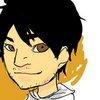
Ryo Yamamoto
9,165 PointsThank you all for your comments! I'll try coding and use different set of dictionaries, too.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThat's a much nicer solution! I like one-liners.
Steven Parker
231,007 PointsSteven Parker
231,007 PointsYou can even abbreviate that a bit more, since the length of a dictionary is the same as the number of keys: