Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial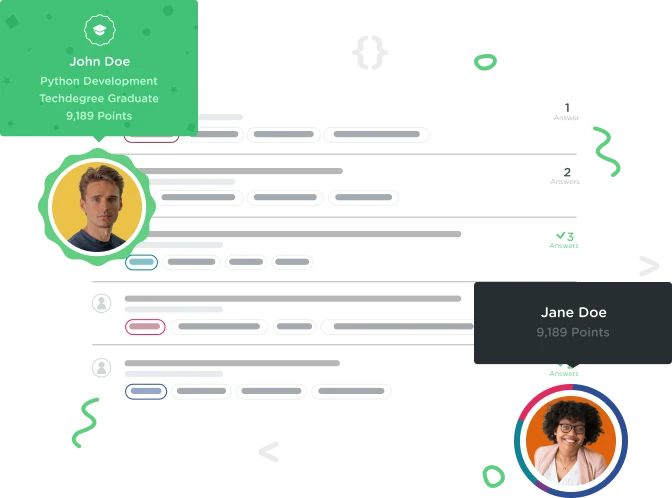
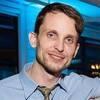
Paul Janson
Courses Plus Student 2,670 Points} while ( ! correctGuess ) ... ?
Intuitively I would think that since ! correctGuess is situated outside the braces, it would be referring to the var defined in line 4: var correctGuess = false;. It would follow that the loop would "do" while correctGuess were !, or true, which obviously doesn't make sense.
Why does ! correctGuess refer to the var inside the do / while function rather than the value of the original var? Is it because even though it's outside the braces, it's still inside the scope of the loop?
7 Answers

Tiff Ting
3,977 PointsThe loop always checks whatever the condition's current boolean value is since the original value may have changed since the last run through the loop. Here are a couple of scenarios of how this particular loop could run. (Keep in mind that the reason why we set correctGuess
to false
before we start looping is because the user cannot have answered correctly before they ever submit a guess.)
User correctly guesses random number on first try
- Function prompts user for a guess.
- User guesses correctly, which changes the current value of
correctGuess
totrue
. - Loop checks whether
!correctGuess
istrue
. If the condition (not the variablecorrectGuess
) istrue
, the loop will run again. But if the condition isfalse
, then the function will exit the loop. - Because
!correctGuess
is nowfalse
, the function exits the loop.
User correctly guesses random number on nth try
- Function prompts user for a guess.
- User guesses incorrectly, so the current value of
correctGuess
continues to befalse
. - Loop checks whether
!correctGuess
istrue
. Again, if the condition (not the variablecorrectGuess
) istrue
, the loop will run again. But if the condition isfalse
, then the function will exit the loop. - Because
!correctGuess
is stilltrue
, the function steps through the loop again. - Repeat steps 1-4 until the user guesses correctly, which changes the current value of
correctGuess
totrue
. - Now that
!correctGuess
isfalse
, the function exits the loop.
If the not operator is throwing you off, you can use the condition correctGuess === false
, which will yield the same result.
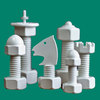
Steven Parker
241,955 PointsYou are correct that the variable is available to the scope of the loop. But the "!" character is the "NOT" operator, so "while (! correctGuess)
" means to loop while "correctGuess" is not true (or while it is false).
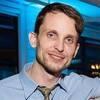
Paul Janson
Courses Plus Student 2,670 PointsStill confused.. isn't the assigned value of correctGuess false? The variable was created var correctGuess = false;. It seems the "NOT" operator would make ! correctGuess loop while correctGuess is not false (or while it is true).
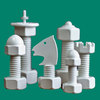
Steven Parker
241,955 PointsThe "NOT" operator doesn't cause the value to be checked against what it was originally assigned, it causes it to be checked for "not true" (or "false"):
while ( correctGuess ) // this will loop while "correctGuess" is true
while ( !correctGuess ) // thils will loop while "correctGuess" is NOT true (while false)

Tiff Ting
3,977 PointsBoth while and do-while loops execute while the supplied condition is true. Since correctGuess
is initially assigned the value of false
, !correctGuess
evaluates to true
until the user enters a guess that matches the random number, at which point correctGuess
is set to true
inside the loop, and !correctGuess
evaluates to false
, which causes the program to exit the loop.
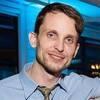
Paul Janson
Courses Plus Student 2,670 PointsI'm on the verge of understanding...
1st off, it seems like there's some discrepancy between Steven and Tiff's answers- does the NOT operator cause the variable to be checked against the original assignment or no?
Assuming it does, my confusion is this- Tiff says,
Both while and do-while loops execute while the supplied condition is true. Since correctGuess is initially assigned the value of false, !correctGuess evaluates to true.
This is where I get lost. If the loop will run until correctGuess is true, and correctGuess is assigned a false value, wouldn't !correctGuess be true and stop the loop before it even gets started?
(For reference, a screenshot of this problem can be seen here- https://imgur.com/a/26Vt3)
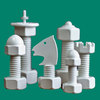
Steven Parker
241,955 PointsThe "NOT" operator only gives the opposite of the current value. Variables don't "remember" what value was originally assigned to them.
And you are correct that if "correctGuess" is assigned a false value, then "!correctGuess" will be true. But this does not stop the loop, instead it allows the loop to repeat. The loop will only stop when "!correctGuess" is false (meaning just "correctGuess" is true).
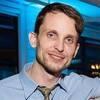
Paul Janson
Courses Plus Student 2,670 PointsOk... so how is the current value of the variable determined if not by what was originally assigned? Is it redefined within the do/while statement? Does this mean that the content associated with the 'while' portion is operating within the scope of the do/while statement, even though it falls outside the braces? If so, here's a stupid question- what's the point in assigning the original value in the first place?
Not trying to be dense, thanks for your patience.
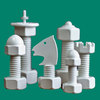
Steven Parker
241,955 PointsAssigning the initial value is done because inside the loop the value is only set to make it "true". So until that happens, you want the value to be "false".
Steven Parker
241,955 PointsSteven Parker
241,955 PointscorrectGuess === false
" will only yield the same result if correctGuess is a boolean (which is the case here). If it were a different type that test would never pass but "!correctGuess
" still might.