Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial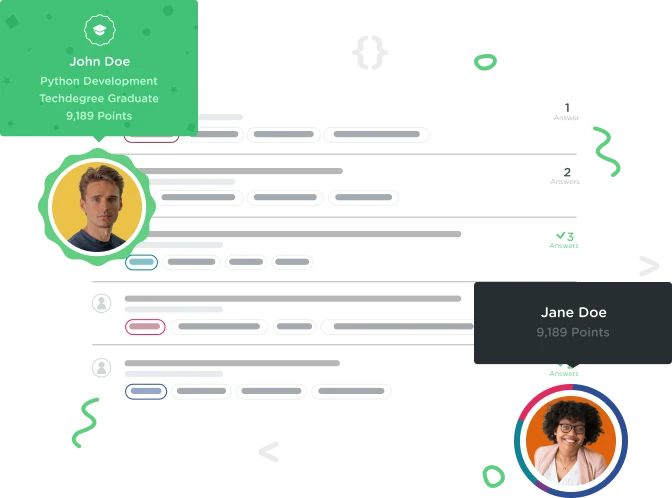

Jonathan Shedd
1,921 PointsA KeyError?
I need to create a function that takes in a string and list of dictionaries and will then proceed to return a new list that uses the format() function to unpack the dictionaries and integrate them into the string. However, I know that there is something wrong with this code and I keep getting a KeyError. All help is appreciated! :)
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
for item in dicts:
return(list(string.format(dicts)))
1 Answer
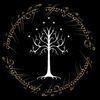
jacinator
11,936 PointsHere's two functions that will work. The first is a bit simpler, the second is shorter and more Pythonic if you are familiar with list comprehension. I can't remember if that's later or earlier in the course. You're getting the KeyError because you aren't unpacking the dictionary into format
so format
is getting a dictionary instead of keyword arguments to feed into the sentence. The other bug that you have is the return inside of the forloop. That won't work for you, as it will only return the first item in the loop.
def string_factory(dictionaries, string):
return_list = []
for dictionary in dictionaries:
return_list.append(string.format(**dictionary))
return return_list
# List comprehension
def string_factory(dictionaries, string):
return [string.format(**dictionary) for dictionary in dictionaries]
Jonathan Shedd
1,921 PointsJonathan Shedd
1,921 PointsI haven't quite gotten to list comprehensions yet. But I understand what I was doing wrong now. Thanks!