Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial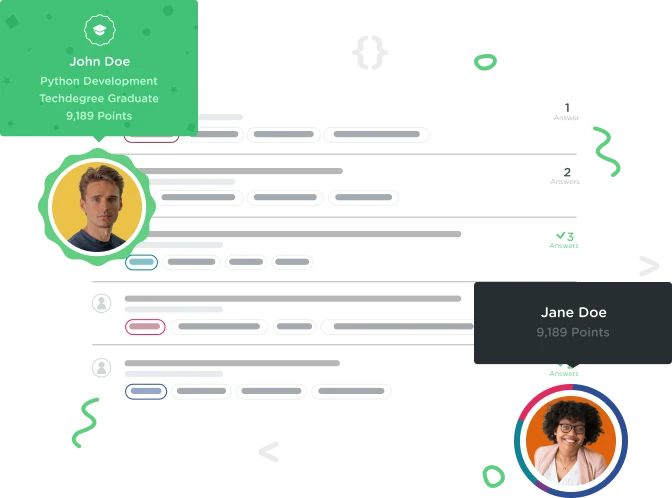

Matt Staten
1,630 PointsA more "pythonic" approach to solving this challenge?
I have passed the "Teacher Stats" challenge using the code attached to this question, but I can't help but wonder if there's a more "pythonic" way to achieve the same result.
For instance, most_classes() and my_dict() both have loops within loops, which is a fairly poor algorithmic technique. If we were using larger datasets, these would run slowly.
My understanding of Python is that things should be done with the fewest system calls possible, aiming for system efficiency over necessary code readability. Am I mistaken in this understanding?
def most_classes(my_dict):
max_count = 0
busiest_teacher = ''
for key in my_dict:
if len(my_dict[key]) > max_count:
max_count = len(my_dict[key])
busiest_teacher = key
continue
else:
continue
return busiest_teacher
def num_teachers(my_dict):
return len(my_dict)
def stats(my_dict):
list_of_teachers = []
for key in my_dict:
my_str = [key, len(my_dict[key])]
list_of_teachers.append(my_str)
return list_of_teachers
def courses(my_dict):
list_of_classes = []
for key in my_dict:
for value in my_dict[key]:
list_of_classes.append(value)
return list_of_classes
2 Answers
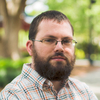
Kenneth Love
Treehouse Guest TeacherYour code isn't bad Python code at all. You don't need those continue
s (or the else:
clause) but that's effectively a no-op anyway. You could leave off my_str
on the stats()
function and just append the new list directly. And then you can use .extend()
instead of that second for
loop and .append()
in the courses()
function.
Other than those fairly small changes, there's not much to be make "more Pythonic".

Charlie L.
10,120 PointsPython should be clean and clear. Your code does both pretty well. There are ways you could improve it if you wanted to.
For example:
def courses(my_dict):
list_of_classes = []
for key in my_dict:
for value in my_dict[key]:
list_of_classes.append(value)
return list_of_classes
Could be changed to:
def courses(my_dict):
list_of_classes = []
for key, value in my_dict:
list_of_classes.append(value)
return list_of_classes
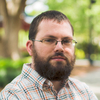
Kenneth Love
Treehouse Guest TeacherI think you mean .extend()
not .append()
. Using .append()
will produce a list of lists.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherAlso, Python is all about readability. I'd rather have code I can read and follow the first time through than code that makes 1 or 2 fewer loops or function calls. The efficiency comes in at the end, when it matters most. Remember, we're gonna compile down to byte code anyway, so cutting out that function probably saves you less than a millisecond in actual execution time. But how much time does it cost the next person (yourself included) that reads the code?