Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial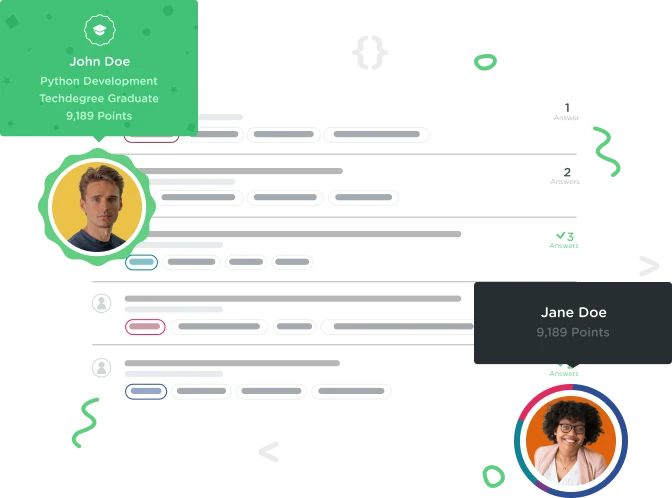

dlpuxdzztg
8,243 PointsA question about lists.
Hello.
I was wondering if there was a way to make a list consisting of more than one object. For example, is it possible to make a list of strings and ints combined? Or if you have a day and hour object, can you make a list consisting of days and hours?
Thanks!
2 Answers

Ulan Assanoff
9,922 PointsIndeed, list only accepts one type parameter. But it can contain different objects as in our case all objects which inherits from Object class You can add Strings, integers and so on. But you cannot do something like this explicitly List<String,Integer> list=new ArrayList<>(); Instead you need to create some custom class which contains some member variables with type of String, Integer and so on

Ulan Assanoff
9,922 PointsThis is an example of custom class which contains different type of fields:
public class Year {
public Year(int day, String hour,String monthName) {
this.day = day;
this.hour = hour;
this.monthName = monthName;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public String getHour() {
return hour;
}
public void setHour(String hour) {
this.hour = hour;
}
public String getMonthName() {
return monthName;
}
public void setMonthName(String monthName) {
this.monthName = monthName;
}
private String monthName;
private int day;
private String hour;
}
Here we can declare our List:
html import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Year> yearList = new ArrayList<Year>();
yearList.add(new Year(29,"09:22 AM","July"));
}
}
Its possible to use List with Object type, but you need to be careful while retrieving objects from List
List<Object> objectList = new ArrayList<Object>();
objectList.add(new Year(29,"09:22 AM","July"));
objectList.add(new String("Hello"));
objectList.add(new Integer(19));
objectList.add(new ArrayList<>());
Ulan Assanoff
9,922 PointsUlan Assanoff
9,922 PointsHi Diego! There are several ways of doing that, for instance you can create list with type Object like below List<Object> list = new ArrayList <Object>(); Another way is to create you own custom Model class which contains some parameters like Strings,Integers whatever you want. Then create a list like below List<MyCustomModelClass> list = new ArrayList<MyCustomModelClass>();