Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial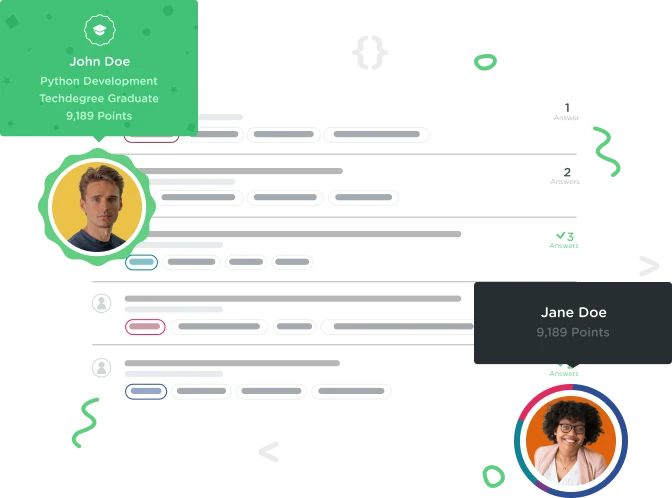
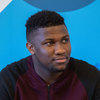
Mohammed Jammeh
37,463 PointsAccessing a variable inside a function..
Hi
I was wondering, how do you access a variable that is inside a function?
I have a function that generates a random number. The random number is then stored in a variable inside the function. However, when I try to access the random number value outside the function, it returns 'undefined' instead of the actual random number value.
How do I solve this?
Thanks..
4 Answers
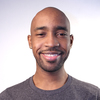
David Tonge
Courses Plus Student 45,640 PointsYou must set function to "return" that variable. Then call the function instead. once you set that variable using the "var" keyword in the function it's gonna be local and you won't be able to access it unless its global (without var keyword) ... but that leads to hoisting problems and running the risk of overwriting that variable later.
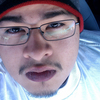
Chyno Deluxe
16,936 PointsAnother option would be to declare the variable outside of the function and overwrite the variable within the function.
var x = 0;
function myFunc(){
x = 2;
}
console.log(x); // returns 0;
myFunc();
console.log(x); // returns 2
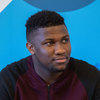
Mohammed Jammeh
37,463 PointsThanks for the reply.
This is the option I am using at the moment but as David said, this is a risky method so I'm kind of contemplating whether to keep it or to try another option.
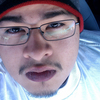
Chyno Deluxe
16,936 PointsYes you want to limit the amount of global variables but if you use this method within another function then it wouldn't be a global variable but a private one.
(function(){
var x = 0;
function myFunc(){
x = 2;
}
console.log(x); // returns 0;
myFunc();
console.log(x); // returns 2
}());
console.log(x) //returns x is not defined
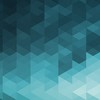
Saleh '
24,986 Pointsfunction generate_random_number_in_a_variable(){
var randomNumber = Math.random();
return randomNumber
}
function return_a_random_number(){
return Math.random();
}
console.log(generate_random_number_in_a_variable());
console.log(return_a_random_number());
/* OUTPUT ==
0.7452114758687594
0.8748426673123808
*/
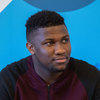
Mohammed Jammeh
37,463 PointsThanks for the replies guys, appreciate it. I will stick with using the global variable and removing the var in front of the variable in my function so that I can access it outside that function.
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsThanks for the reply.
I just want to be able to access the variable in the function, and not call the actual function. However, I am guessing that's impossible but I have tried making the variable global by removing the var keyword. On the other hand, as you have mentioned, this is risky. This is where I am stuck at, I'm thinking of either taking the risk or changing my overall approach just to be able to access the variable.