Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial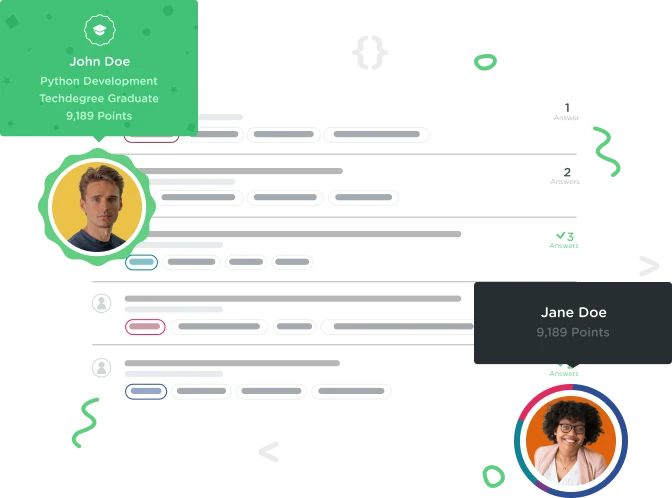
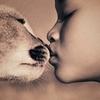
melissakeith
3,766 PointsAccessing arrays nested in objects, why is my last line undefined?
var nameMeanings = {
name: 'Melissa',
languages: [
{greek: [
{melissa: 'honey bee'},
{alexander: 'defending men'}
]
},
{hebrew: [
{shoshana: 'lilly, rose'},
{deborah: 'bee'},
{leah: 'weary'},
{sela: 'rock'}
]
}
]
}
function print(message) {
var div = document.getElementById('output');
div.innerHTML = message;
}
for (prop in nameMeanings) {
console.log(prop); //access 'greek' and 'hebrew'
}
/*for (prop in nameMeanings) {
console.log(prop, ': ', nameMeanings[prop][0]); // access only greek: {melissa: "honey bee"} and hebrew: {shoshana: "lilly, rose}
}*/
//var message = '<p>Hello my name is ' + nameMeanings.name + ' and it means ' + nameMeanings.greek[0].melissa + '.' + '</p>';
//print(message);
console.log(nameMeanings.languages[1][0]); // undefined
1 Answer

efewafewa
21,825 PointsThe problem is that you are jumping from an array to an object.
Let's break it down...
You have a 'languages' array inside your nameMeanings object.
nameMeanings.languages[1];
this gives you...
{hebrew: [
{shoshana: 'lilly, rose'},
{deborah: 'bee'},
{leah: 'weary'},
{sela: 'rock'}
]
}
Now you have an object. You can't access an object by index. Just remember, if it's wrapped in [] its an array, and if it's wrapped in {} it's an object.
An object can't be accessed by it's index (integer), it has a key -> value pair. In this case, what you need is the key of 'hebrew', which has a value of the array that you see under it.
so...
nameMeanings.languages[1].hebrew;
Gives us an array of objects. SO, we must to go deeper still...
nameMeanings.languages[1].hebrew[0];
...will give us the object...
{showhana: 'lilly, rose'}
...to access lilly rose you will hit...
nameMeanings.languages[1]['hebrew'][0].showshana;
which will give you...
lilly, rose
This is the solution, but also a question. This isn't a great way to organize your code, because you aren't using the advantages that arrays and objects give us.
Arrays make it easy to iterate though keys and values incrementally, while objects give us the ability to name a key, and get the value associated with the key, in terms that humans can understand easily.
Don't sweat it though, it will come naturally after some practice.
Let me know if this works for you!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 Pointschanged comment to answer