Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial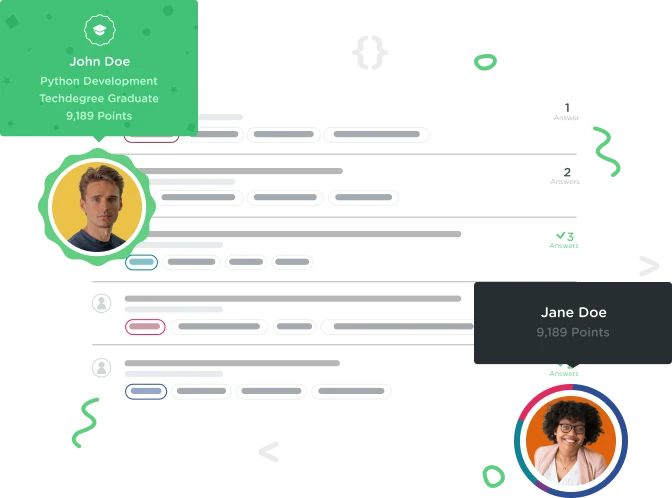

Joshua Whisenhunt
4,266 PointsAccessing the unordered list "rainbow"
I cannot figure out which tag to use for this challenge. I think I should be using document.querySelectorAll() with some combination of 'rainbow' and an attached tag inside the parenthesis.
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
let listItems = document.querySelectorAll('[id=rainbow]');
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
2 Answers

andren
28,558 PointsIn CSS there is a dedicated id selector, you just have to use a #
sign and the name of the id like this #rainbow
to select an element with the id of rainbow
. Though that is not enough to solve this challenge, you have to select the li
elements that are nested within the ul
with the rainbow
id.
That can be done using a descendant selector, with a descendant selector you can specify multiple selectors (id, class or tag) separated by a space. The last element will be selected but only if if is nested within the other element specified.
For example if let's say I wanted to select an a
(anchor) link element found within an element with an id of example
the selector would look like this:
#example a
The querySelectorAll method allows you to use any valid CSS selector so using the above example as a template you should be able to figure out the code to solve this task.

Redweb FED
15,517 PointsHey, Josh
querySelectorAll won't help you out that much here considering its used for elements with classes rather than ID's.
However because you are using an ID you can use this.
let listItem = document.getElementById('rainbow');
However this will only return one item the ul which in your case is not what you want. You need the li's for you for loop, so you are going to need something that returns the collection of li's which is where querySelectorAll would work. however you aren't using any classes for you li elements. This means there has to be another way to get the li's which can be simply done by referencing the children elements of the ID rainbow.
let listItem = document.getElementById('rainbow').children;
This should hopefully solve your problem I made a working example which you can find here. http://codepen.io/Adam_Rusty/pen/dNOpzL

andren
28,558 PointsAdam Russell: You are mistaken about how querySelectorAll
works. It is in no way limited to selecting elements based on classes, it is in fact the most versatile DOM selector function in plain JavaScript.
It accepts any valid CSS selector, meaning that you can select elements not only based on ids, classes and tags but also based on more complex selectors like descendant selectors, attribute selectors, adjacent sibling selectors and so on.
It's not without reason that this challenge directly follows the video where the querySelector
and querySelectorAll
functions were introduced.
To demonstrate this fact I'll provide the solution to the challenge below, using querySelectorAll
with a descendant selector:
let listItems = document.querySelectorAll('#rainbow li');
That line of code will select li
elements nested within the element with the rainbow id.