Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial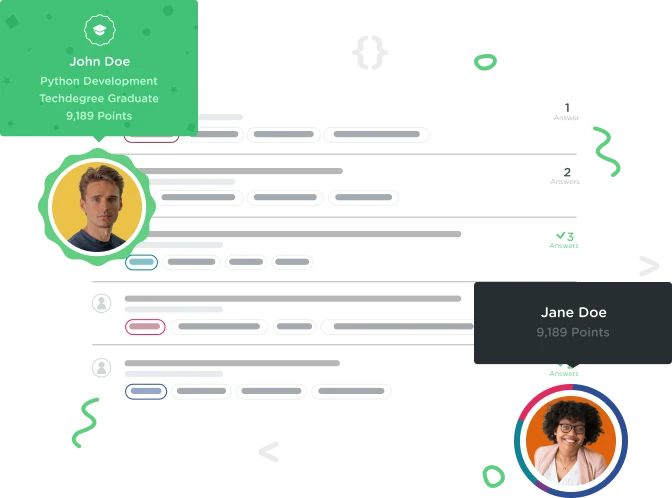

Mason Goetz
5,048 PointsActionView::Template::Error: undefined local variable or method 'todo_item'
I can't find where I defined, or attempted to define, the 'todo_item' locally aside from the let!
statement in the edit_spec.rb. Here is the error message:
$ bin/rspec --format=documentation spec/features/todo_items/edit_spec.rb
Editing todo items
is successful with valid content (FAILED - 1)
Failures:
1) Editing todo items is successful with valid content
Failure/Error: click_link "List Items"
ActionView::Template::Error:
undefined local variable or method `todo_item' for #<#<Class:0x007fe625b18df8>:0x007fe625b182b8>
# ./app/views/todo_items/index.html.erb:6:in `block in _app_views_todo_items_index_html_erb__4514641199172162609_70313225786820'
# ./app/views/todo_items/index.html.erb:5:in `_app_views_todo_items_index_html_erb__4514641199172162609_70313225786820'
# ./spec/features/todo_items/edit_spec.rb:10:in `block in visit_todo_list'
# ./spec/features/todo_items/edit_spec.rb:9:in `visit_todo_list'
# ./spec/features/todo_items/edit_spec.rb:15:in `block (2 levels) in <top (required)>'
and here is my edit_spec.rb file:
require 'spec_helper'
describe "Editing todo items" do
let!(:todo_list) { TodoList.create(title: "Grocery LIst", description: "Groceries")}
let!(:todo_item) { todo_list.todo_items.create(content: "Milk") }
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
within("#todo_item_#{todo_item.id}") do
click_link "Edit"
end
fill_in "Content", with: "Lots of Milk"
click_button "Add It To The List"
expect(page).to have_content("Saved todo list item.")
todo_item.reload
expect(todo_item.title).to eq("Lots of Milk")
end
end
Thanks in advance for the help.
2 Answers

Mason Goetz
5,048 PointsUpon changing that line to this:
within "#todo_list_todo_#{list.id}" do
I get this error message:
Failures:
1) Editing todo items is successful with valid content
Failure/Error: within "#todo_list_todo_#{list.id}" do
Capybara::ElementNotFound:
Unable to find css "#todo_list_todo_1"
# ./spec/features/todo_items/edit_spec.rb:9:in `visit_todo_list'
# ./spec/features/todo_items/edit_spec.rb:15:in `block (2 levels) in <top (required)>'
which isn't an issue that Jason Seifer runs into during the video. He also has the same code on that line that I originally did. Any other ideas?
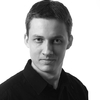
Maciej Czuchnowski
36,441 PointsThis line seems wrong, take a look at it:
within "#todo_list_#{list.id}" do
a todo_
is missing

Product Team
6,835 Points"list" refers to the parameter brought in here: def visit_todo_list(list)
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsThere can be many reasons. Apparently an object with dom.id wa snot created. You'd nee dto show more code. The whle view, controller and model.
Mason Goetz
5,048 PointsMason Goetz
5,048 PointsK. Here is my todo_items_controller.rb:
Here is my todo_item model:
Here is my todo_list model:
And, after switching things back to the code posted in the original question, here is the full error after testing:
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsCan't see anything obiously wrong. Please try changing the last method in the items controller to this:
params.require(:todo_item).permit(:content)
If that doesn't help, you can publish the code on github and I'll try running it locally on my machine.