Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial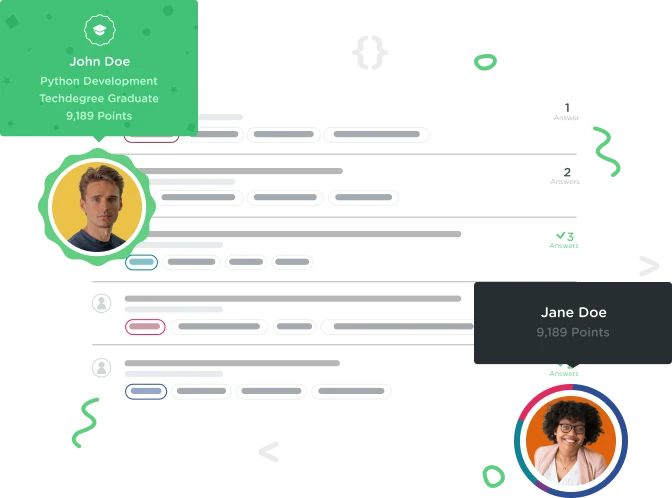
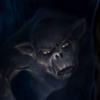
adrastos
8,305 PointsAdd sound to Interactive Story App
Hello all,
I would like to add sound on each different page of the Interactive Story App, can someone please guide me?
Thanks!
package net.tbk.alfavitarimythologias.model;
public class Page {
private int mImageId;
private String mText;
private Choice mChoice1;
private Choice mChoice2;
private int mSound;
private boolean mIsFinal = false;
public Page(int imageId, String text, Choice choice1, Choice choice2, int sound) {
mImageId = imageId;
mText = text;
mChoice1 = choice1;
mChoice2 = choice2;
mSound = sound;
}
public boolean isFinal() {
return mIsFinal;
}
public void setFinal(boolean aFinal) {
mIsFinal = aFinal;
}
public Page(int image, String text, Choice choice1 ) {
mImageId = image;
mText = text;
mChoice1 = choice1;
mChoice2 = null;
mIsFinal = true;
}
public int getImageId() {
return mImageId;
}
public void setImageId(int id){
mImageId = id;
}
public String getText() {
return mText;
}
public void setText(String text) {
mText = text;
}
public Choice getChoice1() {
return mChoice1;
}
public void setChoice1(Choice choice1) {
mChoice1 = choice1;
}
public Choice getChoice2() {
return mChoice2;
}
public void setChoice2(Choice choice2) {
mChoice2 = choice2;
}
public int getSound() {
return mSound;
}
public void setSound(int sound) {
mSound = sound;
}
}
package net.tbk.alfavitarimythologias.model;
import net.tbk.alfavitarimythologias.R;
public class Story {
private Page[] mPages;
public Story(){
mPages = new Page[25];
mPages[0] = new Page(
R.drawable.letter_alpha,
"Αα\n" +
"\n" +
"Αθήνα πόλη της Αθηνάς\n" +
"και όχι του Ποσειδώνα\n" +
"με καμάρι σου την Ακρόπολη\n" +
"και τον Παρθενώνα",
new Choice("Αα", 0),
new Choice("Ββ >", 1),
R.raw.audio1);
Now I know I am missing something...
1 Answer

Ben Jakuben
Treehouse TeacherHi Ilias, this looks good for linking a sound to a page. Are you attempting to use the MediaPlayer class in your Activity somewhere?
If it helps, here is a video from an old archived course about using MediaPlayer (the code still works the same): Adding a Sound