Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial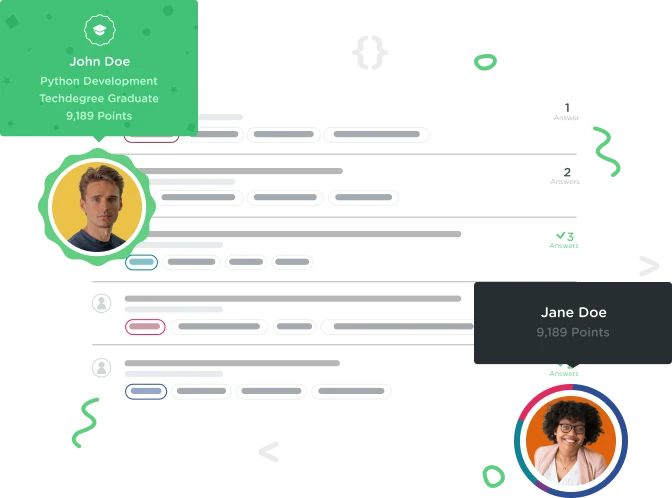

Martin Mundondo
14,355 PointsAdd the code that will allow all Test methods to use the same Artwork object. The Artwork class requires single array pa
Add the code that will allow all Test methods to use the same Artwork object. The Artwork class requires single array parameter. Use the following array of data
$data = [ 'title' => 'mona lisa', 'artist' => 'leonardo da vinci', 'century' => '15th century', 'movement' => 'renaissance', 'location' => 'the louvre (paris, france)', 'type' => 'painting', 'medium' => 'oil on canvas' ];
1 Answer

Aditya Prakarsa
39,803 PointsYou need to set what functions have checked on test. For bottom code, you can set new check get data from array, or you can just check if title same between data array and title on the object. Hope thats help.
<?php
use PHPUnit\Framework\TestCase;
class ArtworkTest extends TestCase
{
// add your code here
protected $artwork;
protected function setUp(): void
{
$data = [
'title' => 'mona lisa',
'artist' => 'leonardo da vinci',
'century' => '15th century',
'movement' => 'renaissance',
'location' => 'the louvre (paris, france)',
'type' => 'painting',
'medium' => 'oil on canvas'
];
$this->artwork = new artwork("Mona Lisa");
$this->artwork->setLocation("Louvre");
}
/** @test */
function hasTitle()
{
$this->assertEquals(
"Mona Lisa",
$this->artwork->getTitle()
);
}
/** @test */
function isAtTheLouvre()
{
$this->assertStringContainsStringIgnoringCase(
'Louvre',
$this->artwork->getLocation()
);
}
//more tests
/** @test */
function sameTitle()
{
$this->assertEquals(
$artwork,
$this->data->title
);
}
}
Mohammed Jammeh
37,463 PointsMohammed Jammeh
37,463 PointsI know this is a bit late but try the code below. I struggled with this task too, it's to get confused on what you actually need to do.