Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial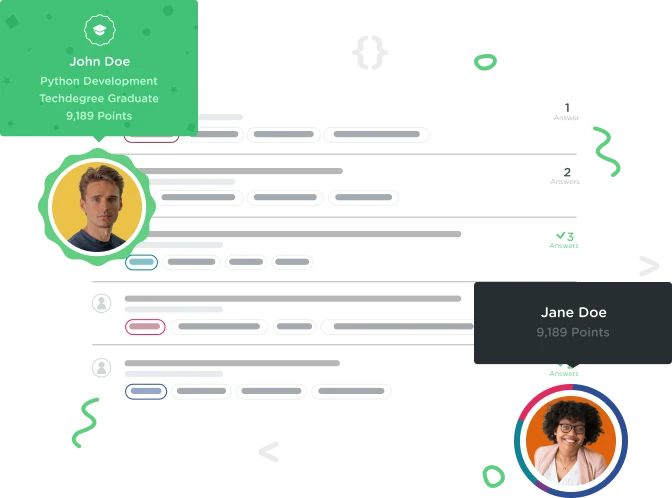
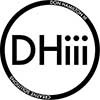
Don Hamilton III
31,828 PointsAdding an error clause?
Hey everyone. I'm trying to take this a step further and add a clause that will be activated if the user puts in a name that isn't on the list. I'm not sure I understand why what I have isn't working. Can someone advise?
Code is posted below and clause is between comment
var message = '';
var student;
var search;
//ERRORS
var noSuchStudent = '<h2>There is no student by that name, please try again.</h2>';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt('Enter a students name to search for them. For a list of all students, type "list". To stop searching, simply type "quit".');
if (search.toLowerCase() === "quit") {
break;
}
// START ERROR CODE
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if (!student.name.toLowerCase().indexOf(search.toLowerCase())) {
message = noSuchStudent;
}
}
// END ERROR CODE
if (search.toLowerCase() === "list") {
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
message += '<p>Points: ' + student.points + '</p>';
}
} else {
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
message += '<p>Points: ' + student.points + '</p>';
}
}
}
print(message);
message = '';
}
1 Answer
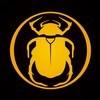
rydavim
18,814 PointsIt seems like the way you have it set up now, your if clause is always going to trigger repeatedly even when the name matches.
It might help if you added a console.log() to your code so you can see the calls as they happen, and what they are returning. If you take a look at the log after searching for a student, you'll see why it's a bit wonky.
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
// Add a console.log to print out the boolean of what you're about to call.
console.log(!student.name.toLowerCase().indexOf(search.toLowerCase()));
if (!student.name.toLowerCase().indexOf(search.toLowerCase())) {
message = noSuchStudent;
}
}
An admittedly lazy way to do this is set message to noSuchStudent if your message is empty after searching your array.
if (message == '') {
message = noSuchStudent;
}
A better way would be to take what you're doing and make it a function.
// Using your code from printing out the student you could do...
function doesStudentExist() { // New function at the top with your other function.
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ) {
return true;
}
}
return false;
}
// And then your error code section could be...
// START ERROR CODE
if (doesStudentExist() == false) {
message = noSuchStudent;
}
// END ERROR CODE
Eventually you'll probably want to trim this down to keep it DRY (Don't Repeat Yourself) using functions so you can do the search and then either print the student, or the no student messages depending on what your search finds.
Hopefully that's helpful and makes sense. Let me know if you have any questions!
Don Hamilton III
31,828 PointsDon Hamilton III
31,828 PointsThat makes a lot of sense. Thanks for the response.