Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial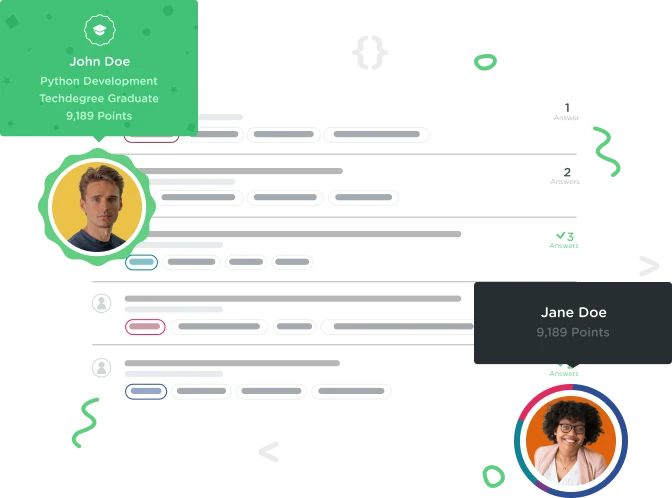
Kirby Abogaa
3,058 PointsAfter 2 hours...FINALLY!!
After 2 hours of brain crunching...I finally got a working program in this challenge which I would like to share...
I feel like the codes are very redundant but, there''s not much of an option since these are the only things covered so far...
Hope this could help someone!
<script type="javascript">
//variables
var serial = 1;
var answer;
var correct = 0;
var medal;
var correctAnswers = "You nailed " + correct + " out of 5 correct answers.";
//Questions
var _q1 = "What is 3 + 3?";
var _q2 = "What is the color of a banana?";
var _q3 = "What is the shape of a full moon?";
var _q4 = "Again, what is 3 x 3?"; //hopefully to confuse the player LOL
var _q5 = "If my age was twice the age of my brother when i was 4 years old, how old will he be when I turn 50?";
//Medal
var gold = "Congratulations! You have earned yourself a GOLD MEDAL.";
var silver = "Congratulations! You have earned yourself a SILVER MEDAL.";
var bronze = "Congratulations! You have earned yourself a BRONZE MEDAL.";
var eggs = "You got eggs! You need to study.";
//Program start
alert("Welcome to The Ultimate Quiz Challenge!");
alert("Question " + serial + " out of 5.");
//for first question
answer = prompt( _q1 + " " + correctAnswers);
if (parseInt(answer) === 6 || answer.toUpperCase() === "SIX" ) {
correct += 1;
serial += 1 ;
alert("Question " + serial + " out of 5.");
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
answer = prompt( _q2 + " " + correctAnswers);
} else {
serial += 1 ;
alert("Question " + serial + " out of 5.");
answer = prompt( _q2 + " " + correctAnswers);
} //end if
//for second question
if (answer.toUpperCase() === "YELLOW" ) {
correct += 1;
serial += 1 ;
alert("Question " + serial + " out of 5.");
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
answer = prompt( _q3 + " " + correctAnswers);
} else {
serial += 1 ;
alert("Question " + serial + " out of 5.");
answer = prompt( _q3 + " " + correctAnswers);
} //end if
//for third question
if (answer.toUpperCase() === "ROUND" || answer.toUpperCase() === "CIRCLE") {
correct += 1;
serial += 1 ;
alert("Question " + serial + " out of 5.");
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
answer = prompt( _q4 + " " + correctAnswers);
} else {
serial += 1 ;
alert("Question " + serial + " out of 5.");
answer = prompt( _q4 + " " + correctAnswers);
} //end if
//for fourth question
if (parseInt(answer) === 9 || answer.toUpperCase() === "NINE" ) {
correct += 1;
serial += 1 ;
alert("Question " + serial + " out of 5.");
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
answer = prompt( _q5 + " " + correctAnswers);
} else {
serial += 1 ;
alert("Question " + serial + " out of 5.");
answer = prompt( _q5 + " " + correctAnswers);
} //end if
//for the fifth question
if (parseInt(answer) === 48 || answer.toUpperCase() === "FORTYEIGHT" || answer.toUpperCase() === "FORTY EIGHT" || answer.toUpperCase() === "FORTY-EIGHT") {
correct += 1;
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
alert(correctAnswers);
} else {
correctAnswers = "You nailed " + correct + " out of 5 correct answer(s).";
alert(correctAnswers);
} //end if
//alert the rewards
if (correct === 5) {
alert(gold);
}else if (correct <= 4 && correct >= 3 ) {
alert(silver);
}else if (correct <= 2 && correct >= 1 ) {
alert(bronze);
}else if (correct === 0) {
alert(eggs);
} //end if
</script>
If anyone has an idea how I can polish this to shorter codes I would gladly appreciate it!
4 Answers
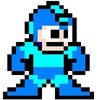
Robert Richey
Courses Plus Student 16,352 PointsGreat job Kirby!
I took your code and tried to make it more generic - I don't present it as the 'best possible way'. We're all learning here :) Feel free to ask if you have any questions - I'll do my best to explain.
function Quiz() {
this.serial = 0;
this.correct = 0;
this.questions = [
{ question : "What is 3 + 3?", answer : 6 },
{ question : "What is the color of a banana?", answer : "yellow" },
{ question : "What is the shape of a full moon?", answer : "circle" },
{ question : "Again, what is 3 x 3?", answer : 9 },
{ question : "If my age was twice the age of my brother when i was 4 years old, how old will he be when I turn 50?", answer : 48 }
];
this.medals = {
gold : "Congratulations! You have earned yourself a GOLD MEDAL.",
silver : "Congratulations! You have earned yourself a SILVER MEDAL.",
bronze : "Congratulations! You have earned yourself a BRONZE MEDAL.",
eggs : "You got eggs! You need to study."
};
}
Quiz.prototype.message = function() {
return "You nailed " + this.correct + " out of " + this.questions.length + " correct answers.";
}
alert("Welcome to The Ultimate Quiz Challenge!");
var quiz = new Quiz();
while (quiz.serial < quiz.questions.length) {
alert("Question " + (quiz.serial + 1) + " out of 5.");
// creating variables to make it easier to deal with current question and answer
var currentQuestion = quiz.questions[quiz.serial].question;
var correctAnswer = quiz.questions[quiz.serial].answer;
var answer = prompt(
currentQuestion + "\n" +
quiz.message() + "\n" +
"Type Quit or Exit to end quiz."
);
// if answer is a string containing a number, change it to a number
if (!isNaN(answer)) { answer = parseInt(answer) }
// if answer is a non-numeric string, change it to lower-case
else { answer = answer.toLowerCase() }
// give the user a way to end the quiz early
if (answer === "quit" || answer === "exit") { break }
if (answer === correctAnswer) { quiz.correct++ }
quiz.serial++;
}
// if the user quit, don't give them a medal!
if (quiz.serial === quiz.questions.length) {
switch (quiz.correct) {
case 5:
alert(quiz.medals.gold);
break;
case 4:
case 3:
alert(quiz.medals.silver);
break;
case 2:
case 1:
alert(quiz.medals.bronze);
break;
case 0:
alert(quiz.medals.eggs);
}
}
Thanks for sharing your accomplishment!
Cheers
Kirby Abogaa
3,058 PointsHi Robert!
Thanks for sharing. You're coding looks a lot clean and readable than mine. Although some of the functions you've used are still unknown to me, I am more excited to learn knowing I could do cool stuff like that in the future.
Cheers!

Karl Taylor
4,043 PointsAt this point in the course, like Kirby Abogaa mentioned. We are yet to be told about functions yet so this will be probably new or confusing to some people. Best to stick to basics before moving on up.
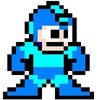
Robert Richey
Courses Plus Student 16,352 PointsI agree with this when the problem hasn't been solved. However, since Kirby did solve it, I felt there was more to gain by showing him something new than to reformat the code into something already familiar.
When I learn the most, it's from reading code that solves a problem in new and interesting ways. For example, suppose you're tasked with adding up all the values of an array whose elements are numbers. We could iterate over each element, adding to sum
the value of each element. Or, we could use reduce()
on the array to achieve the same result.
var numberArray = [1, 2, 3];
var sum = 0;
// reduce takes 4 arguments. I'm only passing in 2, representing the previously returned value
// from the last invocation (if any) and current value
// https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce
sum = numberArray.reduce(function(a, b) {
return a + b
});
console.log(sum); // 6
// or
for (var i = 0; i < numberArray.length; ++i) {
sum += numberArray[i];
}
console.log(sum); // 6
My point is that it's not important to fully understand new or different code. What is important is to understand that we can choose many different ways to solve a problem. Whether or not we use reduce()
or a for
loop, is merely a design decision.
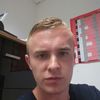
lee F
1,564 Pointswow !! A lot different to what I did!! bloomin heck! Love it that there is so many ways to do things!
var question1 = prompt('What colour is the sky');
var question2 = prompt('How do you spell tie');
var question3 = prompt('How many legs does a dog have');
var question4 = prompt('what colour is black');
var question5 = prompt('How many legs does a dog have if he lost one?');
if (question1.toLowerCase() === ('blue')) {var question1 = parseInt(1);}
else {var question1 = parseInt(0);}
if (question2.toLowerCase() === ('tie')) {var question2 = parseInt(1);}
else {var question2 = parseInt(0);}
if (question3.toLowerCase() === ('4')) {var question3 = parseInt(1);}
else {var question3 = parseInt(0);}
if (question4.toLowerCase() === ('black')) {var question4 = parseInt(1);}
else {var question4 = parseInt(0);}
if (question5.toLowerCase() === ('3')) {var question5 = parseInt(1);}
else {var question5 = parseInt(0);}
var totalscore = parseInt(question1 + question2 + question3 + question4 + question5);
alert('You finished the quiz, you got ' + totalscore +' right!');
if (parseInt(totalscore) < 1) {alert('You suck you didn\'t get a badge');}
if (parseInt(totalscore) < 3 && parseInt(totalscore) > 0) {alert('You got a shitty badge, well done!');}
if (parseInt(totalscore) < 5 && parseInt(totalscore) > 2) {alert('You did, ok!');}
if (parseInt(totalscore) === 5) {alert('YOU ARE THE BEST WAAAHOOOOOO!!!!! PLATINUM BADGE');}
Owen Schebella
4,392 PointsOwen Schebella
4,392 PointsThis kind of bums me out to be honest. I'm at that same point in the course as you I assume and I honestly am stuck with even starting it...I don't know why I find this so damn difficult :(