Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial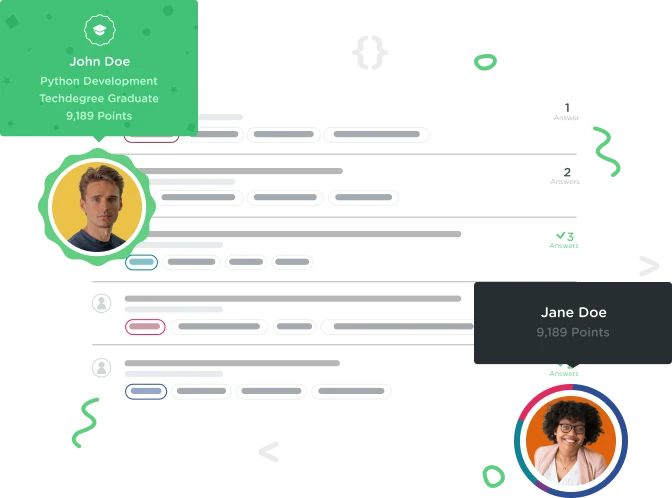

Daven Hietala
8,040 PointsAfter your newly created returnValue function, create a new variable named echo. Set the value of echo to be the results
I have tried and tried and tried to solve this. What am I doing wrong?
function returnValue( 'banana' ) {
var echo = ('banana');
return ( 'banana' );
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
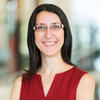
Louise St. Germain
19,424 PointsHi Daven,
The returned value is what comes out of the function, so basically where you have that last line
response_from_echo_function = returnValue( 'string_to_echo' );
It's just saying that it wants that variable name (outside of the function) to be called "echo." Try replacing the last line with this:
var echo = returnValue('hello'); // any string inside the parentheses is fine
If you want, you can further simplify the function itself, since it can just directly return what you just passed to it, without taking the interim step of assigning it to another variable in between. In other words,
function returnValue( string_to_echo ) {
var echo = string_to_echo;
return string_to_echo;
}
// Can be simplified to....
function returnValue( string_to_echo ) {
return string_to_echo; // returns the same thing you sent to it
}
// Either way, don't forget the final line we talked about, where you call the function!
var echo = returnValue('hello');
That should get it working! Sorry I wasn't more clear before. Let me know if that helps!
Louise
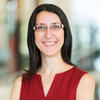
Louise St. Germain
19,424 PointsHi Daven,
The function parameter (i.e., what's in parentheses after the function name) should be a variable name, not an actual string. That way, when the function is called from elsewhere with a string (in this case, the thing to echo back), it has a variable in which to put it.
Also, you'll want to return that echo var. In this case it kind of doesn't matter since echo is going to be the same as the string that got passed in, but that won't usually be the case. Usually, your function will do some sort of process on the input to come up with a different output.
So your code should look something more like this:
// Important: the parameter inside the parentheses should be a variable name
function returnValue( string_to_echo ) {
var echo = string_to_echo;
return echo;
}
Later, you can call the function with a string (like "banana"!) like this:
response_from_echo_function = returnValue( 'banana' );
// You'd send it 'banana'...
//... the returnValue function will put the value 'banana' into its variable string_to_echo
//... then will set its echo variable = string_to_echo (which is 'banana')
//... and it will respond to you with the contents of echo...
//... 'banana'!
Hope this helps! Let me know if there's still any confusion.

Daven Hietala
8,040 PointsThank you so much Louise St. Germain!! I was lost. I had tried making the function parameter without quotation marks and still had no luck. I appreciate the clarification on that part of the problem.
I hadn't even thought of calling the function afterwards. This really helped.
You are awesome, have a wonderful night.
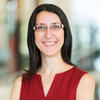
Louise St. Germain
19,424 PointsGreat, I'm glad you got it to work! Have a great night too! :-)

Daven Hietala
8,040 PointsHello again Louise St. Germain.
I thought I understood the answer after our last interaction.
I decided to retry the exercise just to make sure.
I do not know what I am missing here..
function returnValue( string_to_echo ) { var echo = string_to_echo; return string_to_echo; }
response_from_echo_function = returnValue( 'string_to_echo' );
I thought I understood it.
Can you please help me understand what I am doing wrong here?
I keep getting the message "Bummer: Hmmm. It doesn't look like you're storing the returned value in the echo
variable."
I feel like I am definitely storing the returned value in the 'echo' variable.
Please help.