Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial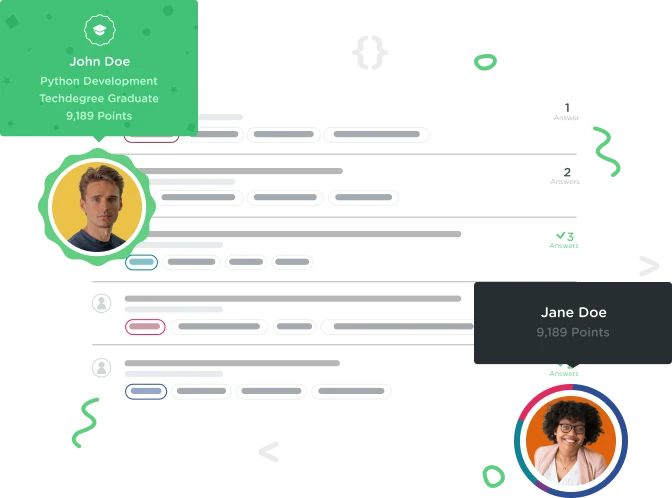

Nicholas Woods
6,260 Points$.ajax method, can someone point me in the right direction.
I tried this this challenge using the $.ajax method, but somewhere I went wrong and I'm having trouble figuring out where.
var url = '../data/rooms.json';
$.ajax(url, {
type : 'GET',
success : function (response) {
var statusHTML2 = '<ul class="rooms">';
$.each(response, function (index, room) {
if (room.available === true) {
statusHTML2 +='<li class="empty">';
} else {
statusHTML2 +='<li class="full">';
}
statusHTML2 += room.room + '</li>';
});
statusHTML2 += '</ul>';
$('#roomList').html(statusHTML2);
}
});
2 Answers
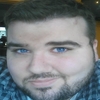
Marcus Parsons
15,719 PointsHey Nicholas,
Be sure that you're using different variables for your JSON request than you used in the employees section of JSON.
You can, however, reuse the same code in both sets of JSON requests if you wrap each request within a function so that the variables are destroyed upon function end:
function getEmployeeData() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i < employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/employees.json');
xhr.send();
}
function getRoomData() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var rooms = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="rooms">';
for (var i = 0; i < rooms.length; i += 1) {
if (rooms[i].available === true) {
statusHTML += '<li class="empty">';
} else {
statusHTML += '<li class="full">';
}
statusHTML += rooms[i].room;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('roomList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/rooms.json');
xhr.send();
}
//Call each function
getEmployeeData();
getRoomData();
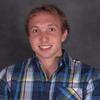
Franklyn Roth
16,770 PointsThis was my result. with the ajax method. the "type" is "GET" by default as per the documentation so it can be omitted. I used a for loop because It's much more common for me to use that then "each()" at work with c#/net.
//get rooms
var roomUrl = "../data/rooms.json";
$.ajax({
url: roomUrl,
dataType: "json",
success: function(response){
var roomStatus = '<ul class="rooms">';
for(var i = 0; i < response.length; i++){
if(response[i].available === false){
roomStatus +='<li class="full">';
}
else {
roomStatus +='<li class="empty">';
}
roomStatus += response[i].room +'</li>';
}
roomStatus +='</ul class="rooms">';
$('#roomList').html(roomStatus);
}
});
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsYea I was using console.log() for all my variables as I find plain JavaScript a lot easier than jQuery and I wasn't sure what data was being sent to them (I need to do this more).
But because I was using $.ajax object method I needed to define the dataType : JSON. When Dave mentioned this method he was using POST not GET and I don't believe he defined dataType (so I guess you only need to define that for incoming data). Either way this has fixed my code. I also moved the url into the $.ajax object as I find it easier to read this way.