Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial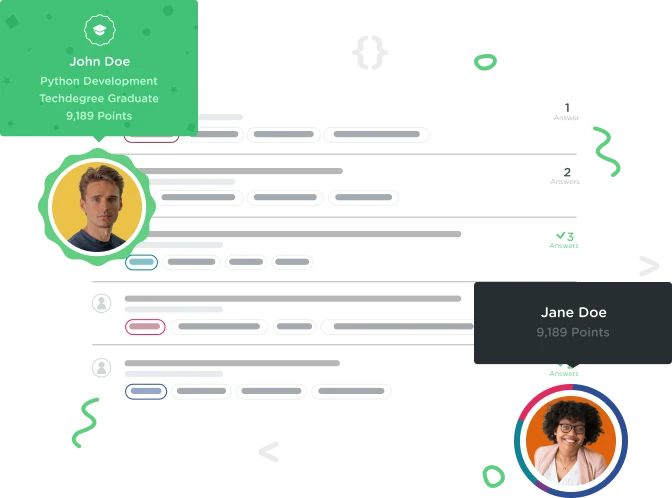
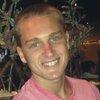
Chandler Tate
3,963 PointsAll of my questions are coming back as incorrect for the user
For the conditional challenge, you're asked to create a five question quiz that keeps track of the user's score and gives them a crown (I used medal in place of this) at the end based upon their score. However, all of the questions in my program return an incorrect answer for the user and I can't figure out what's going wrong! Any help would be greatly appreciated! :)
http://port-80-ywfm1jo78g.treehouse-app.com
// Common variables
var score = 0; var answer = false;
// Beginning of quiz
alert('Welcome to the quiz. There will be five questions in total.');
// Ask user for name
var name = prompt('What is your name?');
// First question
var answerOne = prompt('What does 2 + 2 equal?'); if (parseInt(answerOne) === '4') { answer = true; alert('You answered correctly. 1 out of 5 questions correct.'); score += 1; } else { alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.'); }
// Second question
var answerTwo = prompt('What country is Sydney located in?');
if (answerTwo.toUpperCase() === 'Australia') {
answer = true;
alert('You answered correctly. ' + score + ' out of 5 questions correct.');
score += 1;
} else {
alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.');
}
// Third question
var answerThree = prompt('What sport do the Seattle Seahawks play?');
if (answerThree.toUpperCase() === 'football' || answerThree.toUpperCase() === 'nfl') {
answer = true;
alert('You answered correctly. ' + score + ' out of 5 questions correct.');
score += 1;
} else {
alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.');
}
// Fourth question
var answerFour = prompt('How many ounces are in one cup?'); if (parseInt(answerFour) === '8' || parseInt(answerFour) === 'eight' ) { answer = true; alert('You answered correctly. ' + score + ' out of 5 questions correct.'); score += 1; } else { alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.'); }
// Fifth question
var answerFive = prompt('Where is Surfers Paradise located?'); if (answerFive.toUpperCase() === 'Gold Coast') { answer = true; alert('You answered correctly. ' + score + ' out of 5 questions correct.'); score += 1; } else { alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.'); }
// Quiz report card
document.write('You answered ' + score + ' questions correctly '+ name + '. '); if (score === 5) { document.write('You received a gold medal for answered all five questions correctly!'); } else if (score === 3 || score === 4) { document.write('You received a silver medal for answering at least three questions correctly!'); } else if (score === 1 || score === 2) { document.write('You received a bronze medal for answered at least one questions correctly!'); } else { document.write('You did not receive a medal since 0 questions were anwered correctly.'); }
5 Answers
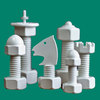
Steven Parker
231,007 PointsFor each question, you've set up the test so that the answer cannot be right:
if (parseInt(answerOne) === '4') -- you're doing a type-sensitive equality test between a number and a string
if (answerTwo.toUpperCase() === 'Australia') -- you forced the answer to uppercase, but compare it against a mixed-case word
if (answerThree.toUpperCase() === 'football' || answerThree.toUpperCase() === 'nfl') -- case mismatch again
if (parseInt(answerFour) === '8' || parseInt(answerFour) === 'eight') -- two type mismatches
if (answerFive.toUpperCase() === 'Gold Coast') -- one more case mismatch
You can easily fix these issues with the following replacement lines:
if (parseInt(answerOne) == 4 || answerOne.toLowerCase() == 'four')
if (answerTwo.toLowerCase() == 'australia')
if (answerThree.toLowerCase() == 'football' || answerThree.toLowerCase() === 'nfl')
if (parseInt(answerFour) == 8 || answerFour.toLowerCase() == 'eight')
if (answerFive.toLowerCase() == 'gold coast')
(Type-sensitive comparisons are not needed here.)
Also, there are 4 places where you do this:
alert('You answered correctly. ' + score + ' out of 5 questions correct.');
score += 1;
You probably want to increment the score before you do the alert.
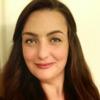
Jennifer Nordell
Treehouse TeacherHere's an example of something I see that's incorrect. And there's several instances of this:
if (answerFive.toUpperCase() === 'Gold Coast')
That evaluation will always be false. Because no matter what I type, if it turns it all to upper case it will never be equal to Gold Coast. For example, if I type in "goLD CoasT" the to uppercase will take that and turn it into "GOLD COAST". So the evaluation needs to be:
if (answerFive.toUpperCase() === 'GOLD COAST')
Try changing those first, and see if something doesn't start working :) But let us know if there are still problems left!
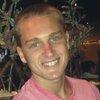
Chandler Tate
3,963 PointsThanks Jennifer! That seemed to have fixed the majority of the questions, but I'm still having issues with a few answer inputs. For example, questionOne still returns an incorrect answer when inputting 4. questionTwo comes back correct, but the score still says 0 out 5 answered correctly and from there on out the counter is all messed up (for the total score). Lastly, inputting 8 as an answer for questionFour comes back incorrect as well :(

Ayaz Hussein
13,348 PointsYou haven't structured your if conditional correctly,
if (answerTwo.toUpperCase() === 'Australia')
doesn't work because the the string is turned to Upper case and it's not equal to the string you wrote, instead you should do it like this:
if (answerTwo.toUpperCase() === 'AUSTRALIA')
and the other question where you parseInt the answer , you have to use an integer and not a string
if (parseInt(answerOne) === '4')
you should've written
if (parseInt(answerOne) === 4)
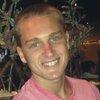
Chandler Tate
3,963 PointsAh, thanks! That was the issue! I had the integers in strings and the string answers weren't capitalized! thanks Ayaz!

Seth Kroger
56,413 PointsLet's take a look at some of your if statements. For you first question you have:
if (parseInt(answerOne) === '4')
Triple-equals can be true only if the two sides are the same type and same value. One side you will always have a number (from parseInt) but the other is a string because of the quote marks. Because the types are different, this if statement will always be false.
if (answerTwo.toUpperCase() === 'Australia')
Using toUpperCase keep in mind if you type in "AuStRaLiA" you'll get "AUSTRAILIA", not "Australia". If you go back over your if statement with these two things in mind, you should be able to get in to work.
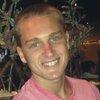
Chandler Tate
3,963 PointsThanks Seth! That cleared things up for me! Everything seems to be working now! :)
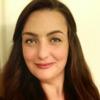
Jennifer Nordell
Treehouse TeacherAyaz Hussein is correct about the strings instead of integers. That being said you could leave out the parseInt and write it as such:
if(answerOne = "4");
This is because whatever is read in from the prompt is a string... even if it's a number.
Also, increment your score before you print the alert. The way you have it now, if you had just gotten the first one correct it will print out that your score is 0 and then change the score to 1.
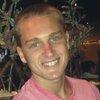
Chandler Tate
3,963 PointsThanks Jennifer! The score incrementing issue completely flew over my head. Couldn't figure out why that wasn't working until now :) haha
Chandler Tate
3,963 PointsChandler Tate
3,963 PointsThat solved both issues including the score counter problem! Thanks Steven! The only issue I'm having now is a string answer to question four that comes back as negative if the user inputs eight. Any ideas as to what could be going wrong here?
// Fourth question
var answerFour = prompt('How many ounces are in one cup?'); if (parseInt(answerFour) === 8 || parseInt(answerFour.toUpperCase) === 'EIGHT' ) { answer = true; score += 1; alert('You answered correctly. ' + score + ' out of 5 questions correct.'); } else { alert('Your answer was incorrect. You have answered ' + score + ' questions correctly.'); }
Steven Parker
231,007 PointsSteven Parker
231,007 PointsI had a typo. I repaired it in the code above.
In your version: if (parseInt(answerFour) === 8 || parseInt(answerFour.toUpperCase) === 'EIGHT' ) -- you apply the toUpperCase method without the parentheses, making it a reference instead of a call. Then you convert it to a number and compare it to the word 'EIGHT'.
Ayaz Hussein
13,348 PointsAyaz Hussein
13,348 Pointsremove the parseInt after ||