Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial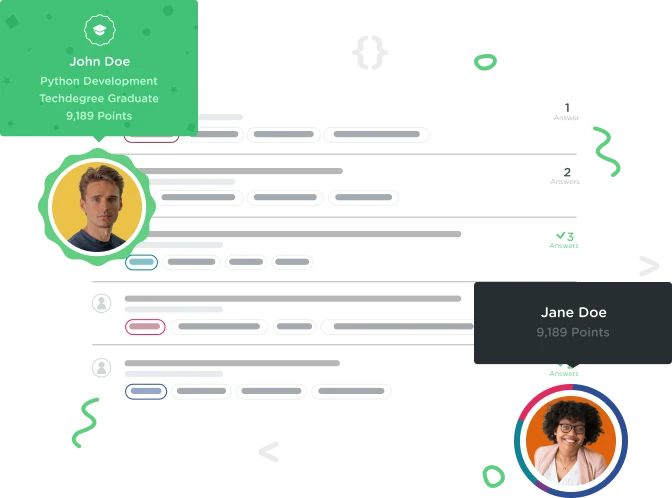

Brandon Harrison
1,283 PointsAm I even on the right track with this decrement code?
I have been stuck on this for a couple of days now and do not know what to do at this point. the task at hand is a bit confusing to me.
import random
start = 5
def even_odd(num):
return not num % 2
while start is True:
if random.randint(1,99) is % 2:
print("{} is even".format(even_odd))
elif random.randint(1.99) not % 2:
print("{} is odd".format(even_odd))
start -= 1
2 Answers
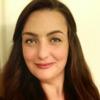
Jennifer Nordell
Treehouse TeacherHi there! Unfortunately, I see a multitude of problems with your code. But that could be because you've worked on it so long that it now no longer resembles what you started with. So I'm going to show you my solution and then walk you through it.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while(start):
randNum = random.randint(1, 99)
if (even_odd(randNum)):
print("{} is even".format(randNum))
else:
print("{} is odd".format(randNum))
start -= 1
Ok so the even_odd function is something that Treehouse provided for us, and we do not do any coding in there. All it 's going to do is return a true if a number is even and false if it's odd. That's it's sole purpose.
Secondly, we set up a variable named start and assign it to 5. This should bring you through the second step.
Now, the first two steps are rather straight-forward. The next step is going to challenge you to think "truthy". Remember, we're going to run this while loop while start is "truthy". The value of start is 5. Any non-zero number is "truthy". But it's not explicitly equal to True. True is "truthy" and 5 is "truthy" and 1093890 is "truthy". But only the first one is exactly equal to True. With your code, the loop will never start. Because 5 is not equal to True.
I set up a variable to get a random number between 1 and 99. Then I send the random number I generated into the even_odd function. If it returns a true, we print out that the number was even. Otherwise, we print out a number was odd message.
Lastly, your start -= 1 is not indented correctly. This will result in you getting far more results than expected as the start number will only decrement if the number is odd. We want this to decrement every time through the loop. When start reaches 0 start will no longer be "truthy". Remember "truthy" can be any non-zero number. But at 0 it becomes "falsey". The loop will then exit.
I hope this helps! Good luck!
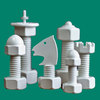
Steven Parker
231,007 PointsIt looks like your sequence of events is a bit confused. Try doing the challenge parts in steps.
- use random.randint(1, 99) to get a random number (and save it somewhere)
- use even_odd to find out if the number is even (you will only use it once)
- there's only two possible cases so you can use a plain "else" for the second one
- when you print, use format to include the number you picked and saved earlier
- be sure to decrement the counter in either case
Brandon Harrison
1,283 PointsBrandon Harrison
1,283 Pointsthank you. I get these complicated questions (at least they're complicated to me) so I keep think I need something complex but then I see it and it is actually very simple.
Eddy Avila
846 PointsEddy Avila
846 PointsI used your code and keep getting a "name 'random' is not defined". I even copied and pasted the damn thing after typing and toying with it a few times. What gives?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherEddy Avila Hi there! I have no idea what the problem is. If i take the code I posted above and paste it in the challenge, it passes all three steps.
Eddy Avila
846 PointsEddy Avila
846 PointsAH! Nevermind. Figured out the issue. Didn't realize my browser refreshed and sent me back to task one. Son of a gun! But thanks!
Eddy Avila
846 PointsEddy Avila
846 PointsBTW, Jennifer Nordell, your step-by-step explanation was great. Let's get you on some videos!
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherEddy Avila well aren't you sweet?
Just glad I can help out every great once in a while 