Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial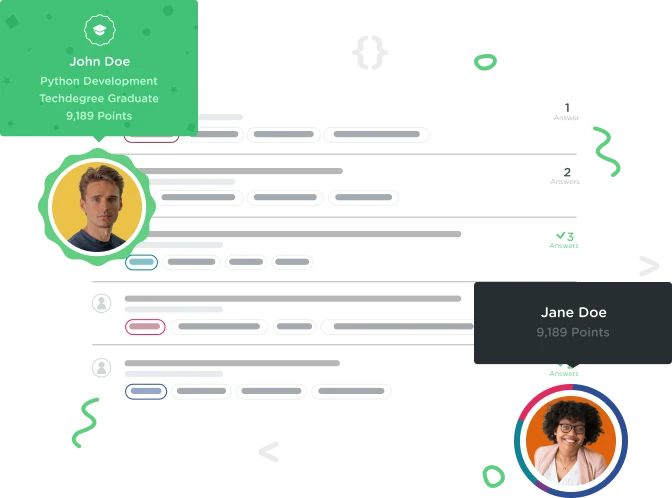
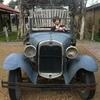
Brendan Moran
14,052 PointsAnother Solution -- Thoughts?
I wanted to show the user both the question and the answer, but for the incorrect answers, I also wanted to show them their answer alongside the correct answer. This required pushing their response to the correct/incorrect answer arrays, along with the question arrays, and the spread operator was necessary there. After that, the toughest part was figuring out a decent way to test if the loop was dealing with correct or incorrect answers. The most straightforward thing I could think to do this was to add a "tag" to the beginning of each array with simply the string value, "correct" or "incorrect", and then test for that in the loop. It was the least hacky way I could think of, trying to keep it relatively easy to read, concise, and dry. It turned out to be a simple solution, but is it OK practice?
nb: I have a function that is creating an entire ol, but there's no reason for that other than kicks and giggles. You could do the same thing with createListItems function.
const questions = [
['How many planets are in the solar system? (Pluto counts!)', '9'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6'],
['What year was JavaScript created?', '1995'],
["What is Robert Plant's middle name?", "Allen"]
];
let correctAnswers = ["correct"];
let wrongAnswers = ["incorrect"];
function createOrderedList(array) {
let listHTML = `<ol>`;
for (let i = 1; i < array.length; i += 1) {
if (array[0] === "correct") {
listHTML += `
<li>
<strong>Q:</strong> ${array[i][0]}<br>
<strong>A:</strong> ${array[i][1]}
</li>`
} else {
listHTML += `
<li>
<strong>Q:</strong> ${array[i][0]}<br>
<strong>A:</strong> ${array[i][1]} <em style="opacity: 0.5">(Your answer: ${array[i][2]})</em>
</li>`
}
}
listHTML += `</ol>`;
return listHTML;
}
for (let i = 0; i < questions.length; i++) {
let question = questions[i][0];
let answer = questions[i][1];
let response = prompt(question);
if ( response.toLowerCase() === answer.toLowerCase() ) {
correctAnswers.push([...questions[i], response]);
} else {
wrongAnswers.push([...questions[i], response]);
}
}
//Display results to the user
document.querySelector('main').innerHTML = `
<h1>You got ${correctAnswers.length - 1} out of ${questions.length} answers right!</h1>
<h2>Correct:</h2>
${createOrderedList(correctAnswers)}
<h2>Incorrect:</h2>
${createOrderedList(wrongAnswers)}
`;
1 Answer

Nasra Iid
Front End Web Development Techdegree Student 9,876 PointsThe easies way I could thing of is to interpolation both question and answer
correct.push (${question} ${answer}
);
}else {
incorrect.push(${question} ${answer}
);
This will print both question and answer