Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial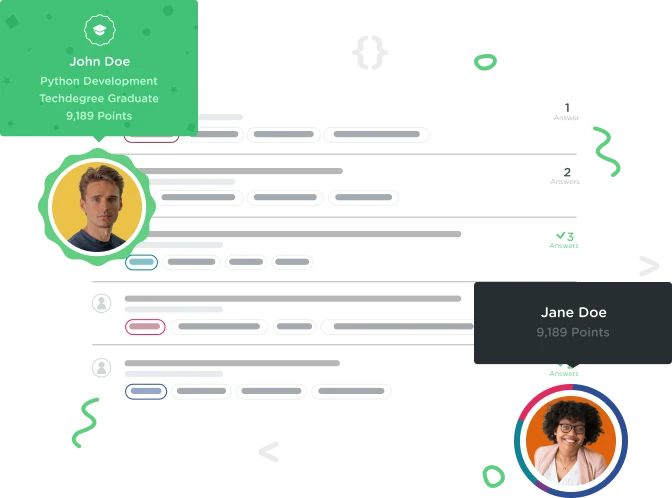
Juan García
10,723 PointsAnother way of solving the challenge
// First we set the variables for the questions and answers
var answer1 = false;
var answer2 = false;
var answer3 = false;
var answer4 = false;
var answer5 = false;
var correctAnswers = 0
var question1 = prompt('Which color is the sky?');
var question2 = prompt("Which company is the creator of the MacBook?");
var question3 = prompt("Who is Luke Skywalker's father?");
var question4 = prompt("What is the name of Link's fairy?");
var question5 = prompt("What is the name of the coldest region in The Elder Scrolls?");
// Then we set the conditions to determine if the answer is true or false
if (question1.toUpperCase() === 'BLUE') {
answer1 = true;
correctAnswers += 1
}
if (question2.toUpperCase() === 'APPLE') {
answer2 = true;
correctAnswers += 1;
}
if (question3.toUpperCase() === 'DARTH VADER') {
answer3 = true;
correctAnswers += 1;
}
if (question4.toUpperCase() === 'NAVI') {
answer4 = true;
correctAnswers += 1;
}
if (question5.toUpperCase() === 'SKYRIM') {
answer5 = true;
correctAnswers += 1;
}
//We then tell the user how many questions he got right
document.write('<p>You answered ' + correctAnswers + ' questions correctly</p>');
//Then we set the conditions to earn the different crowns
if (correctAnswers === 5) {
document.write('<p>You won the golden crown!</p>')
}
if (correctAnswers === 3 || correctAnswers === 4) {
document.write('<p>You won the silver crown!</p>');
}
if (correctAnswers === 1 || correctAnswers === 2) {
document.write('<p>You won the bronze crown</p>')
}
if (correctAnswers === 0) {
document.write('<p>You won no crown. You suck.</p>');
}
Notice that I set the answer var at the beginning, though it has no use.
Also, I set the conditions with equal signs, so I could test the || symbols.
In this case I guess there's no need to use the else if, so I just used several if conditional statements.
Lastly, I noticed that at first my code didn't run, and I had to indent the <Script> code within the HTML to make it work.
1 Answer
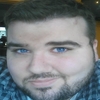
Marcus Parsons
15,719 PointsHey Juan García,
That's a good way of getting the problem done. The only thing I can say about the program itself is that you are missing some semicolons in a few places.
You said that var has no use, but it does. Yes, you can set a variable without using the var keyword, and it will seem to work in just the same fashion as if you set it with the "var" keyword for this case.
However, the difference between not using var and using var comes into play when you start doing functions. The "var" keyword will initialize a variable within the scope of where it is initialized (global or function) whereas if you leave the keyword off, you are either initializing/setting it in the global scope. This will come into play if you have a fairly large program and you have two functions that use the same variable name for different purposes. You have to decide whether the variable should pull from the global scope or be initialized within the function.