Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial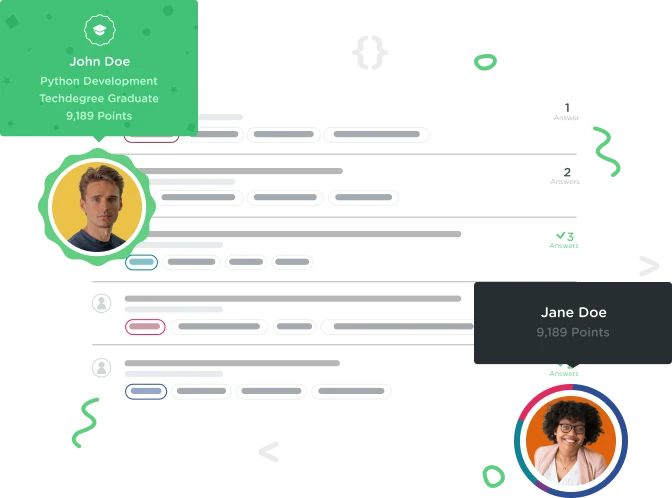

Noe Arzate
Python Web Development Techdegree Student 6,077 PointsAny assistance with failable init code challenge would be greatly appreciated.
I think I understand what they're asking but I'm stuck on what to do next. Any help is greatly appreciated.
Thank you,
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String : String]) {
return nil
}
}
2 Answers
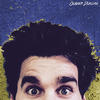
Oliver Duncan
16,642 PointsYou wouldn't initialize a book class with a dictionary, you would initialize it with two mandatory Strings, a title and author, and two optional Strings, price and pubDate. If either mandatory string were undefined, you would want the init to fail and return nil.
EDIT: I checked the challenge. It is asking for you to init with a dictionary. Here's how I would do it:
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String:String]) {
guard let title = dict["title"], let author = dict["author"] else { return nil }
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}

Noe Arzate
Python Web Development Techdegree Student 6,077 PointsI do understand what you're saying but I'm sure how to do that in code. Well, everything I've tried in xcode gives me error messages. Would you mind sharing code snippet of the implementation? Thanks
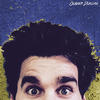
Oliver Duncan
16,642 PointsI edited with my implementation. Moving forward, you should use comments instead of new answers to communicate.
Noe Arzate
Python Web Development Techdegree Student 6,077 PointsNoe Arzate
Python Web Development Techdegree Student 6,077 PointsThat is almost what I had in xcode but I can't get it to work in xcode. For title and author I keep getting error message "variable declared in 'guard' condition is not usable in its condition". The code above worked fine for the code challenge but it will not in xcode. I will need to do more reading up on failable init to understand why it fails in xcode.
Thanks
Oliver Duncan
16,642 PointsOliver Duncan
16,642 PointsI have no idea why the above code wouldn't compile in an Xcode Playground - maybe you don't you have the latest Xcode? Works in mine.
Noe Arzate
Python Web Development Techdegree Student 6,077 PointsNoe Arzate
Python Web Development Techdegree Student 6,077 PointsIt was a missing "}" after the return nil. Usually xcode is very specific about a missing closing brace but not this time. Yes, the code above compiles fine xcode. So basically, if title and author are not present then it returns nil.
Thanks