Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial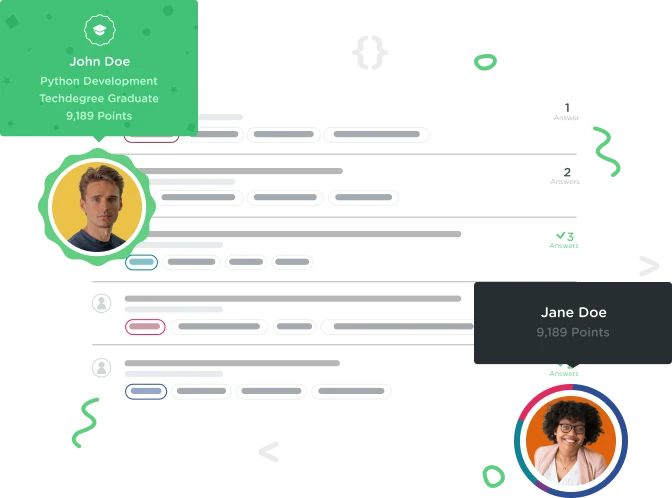

Saqib Ishfaq
13,912 PointsAny suggestions abt my code?
function getRandomNum(upperNum, lowerNum) {
var randomNumGen = Math.floor(Math.random() * (upperNum - lowerNum + 1)) + 1;
return randomNumGen;
}
console.log ( getRandomNum(78, 9) );
console.log ( getRandomNum(100, 1) );
console.log ( getRandomNum(6, 1) );
console.log ( getRandomNum(6, 0) );
9 Answers
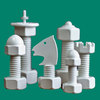
Steven Parker
231,275 PointsAssuming the formula is intended to generate random numbers between the upperNum and lowerNum (inclusively), then the last term should be lowerNum instead of 1.
Optionally, you could eliminate the randomNumGen variable and return the result of calculation directly.
It's probably more conventional to have the lower number argument come first, but it's not an issue as long as it's used consistently (which it is in your sample calls).
And some "best practice" style guides would suggest removing the blank line inside the function, and having the open parenthesis follow a function or method name with no space in between.
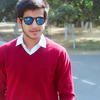
Gaurav Yadav
5,047 PointsWhy are you using upperNum and lowerNum... I mean your code is perfectly fine..but u could just use one value? In other words if u have to explain what does ur function do...what would you say?
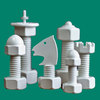
Steven Parker
231,275 PointsI suspect this code is intended to generate a random number within a specified range, which it will do once the error is corrected.

Saqib Ishfaq
13,912 Pointsi thought our purpose of this challenge was to generate a number between two values...thats why i did this:(
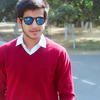
Gaurav Yadav
5,047 PointsBut for that there is no need of lowerNum don't u think? For example in the first console.log the program gives a random number between 0 and 70 both included ...so there was no need of 78 or 9...he could have just provided an upperNum argument of 70 and modified some of his code...But again may be he wanted to do something else...
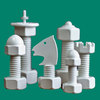
Steven Parker
231,275 PointsBut based on the provided arguments, I would expect that what is required is a random number between 78 and 9 (not 70 and 0).
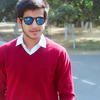
Gaurav Yadav
5,047 Pointsvar randomNumGen = Math.floor(Math.random() * (upperNum - lowerNum + 1)) + 1;
Suppose upperNum = 78 and lowerNum = 9, the term (upperNum - lowerNum + 1) becomes 70. Math.random() * 70 will give a number between 0 and 70, 70 not included, then Math.floor() will give an integer value between 0 and 70, 70 not included, then the program adds 1 and returns the number, So according to me the program gives a value between 0 and 70, both included..Please explain if I am wrong

Saqib Ishfaq
13,912 Pointsyes steven thats what my intention was! is it wrong?

Saqib Ishfaq
13,912 PointsGaurav does it mean when i put "78" and "9" it actually getting a value between "0" and "70" instead?
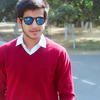
Gaurav Yadav
5,047 Pointsyes, run the code with 78 and 9 and you will see that you will never get a number greater than 70, then try to understand why is it happening, meanwhile I am trying to get the solution of your original problem, u want a random number in the range of upperNum and lowerNum, right?

Saqib Ishfaq
13,912 Pointsyes. that's wot my intention was as per challenge. and i just saw the solution video ,teacher did the same thing passing two arguments , with one change he put lower 1st and then upper. does it make any difference? like in the formula provided if we put lower 1st or upper?
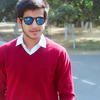
Gaurav Yadav
5,047 Pointsno, it does not make any difference which argument you pass first, but you have to be careful that howsoever you are passing the values, your function declaration should accept parameters in that order. for example if you are passing
getRandomNum(6,0)
you function declaration should be:
function getRandomNum(upperNum, lowerNum){
//your code
}
and not :
function getRandomNum(lowerNum, upperNum){
//your code
}
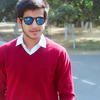
Gaurav Yadav
5,047 PointsDid u understand what is the problem with the current version of your code? Why is it returning a number between 0 and 70?

Saqib Ishfaq
13,912 Pointskind of, coz its adding the "1" in the end.. ...after i saw the solution video ,i have to add lowerNum instead of "1" . dont know why though?
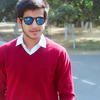
Gaurav Yadav
5,047 Pointsyes, Do this:
- read what does Math.random() function do on Mozilla Developer Network (MDN).
- Understand what does your version of code do ie. why does your code logs out a number between 0 and 70.
- Try to understand what happens when you add lowerNum instead of 1. Don't lose hope..If you still dont understand, leave a comment, i will try to explain further. But, try to do it yourself first

Saqib Ishfaq
13,912 Pointsyeh just read the whole article on it on MDN, make sense now! i was just doing simple maths in my head, n doing same on the code but its the formula in action, which explains on MDN .

Saqib Ishfaq
13,912 PointsDon't see the best answer option on the answer I wanna mark as best
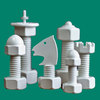
Steven Parker
231,275 PointsTake another look, every answer should have a "best answer" option.
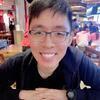
Chris Phua
6,884 Pointsvar upperValue = prompt("Please key in an upper value");
var lowerValue = prompt("Please key in an lower value");
function randNumGenerator(x, y){
var randNum = Math.floor(Math.random() * parseInt(x));
if (randNum >= parseInt(y) && randNum <= parseInt(x)) {
return randNum;
} else {
return randNumGenerator(x, y); /*---> RECURSIVE: call the same function again until it falls into the statement*/
}
}
console.log(randNumGenerator(upperValue, lowerValue));
/*This method of calling is not efficient but it solves the challenge. Hope it helps.*/
Saqib Ishfaq
13,912 PointsSaqib Ishfaq
13,912 Pointssorry i dint undertand the 1st part of ur answer.
Math.floor(Math.random() * (upperNum - lowerNum + lowerNum)) + lowerNum
is that wot u meant? and how would removing randomNumGen be any option, i dont understand! and lastly ,why does my code