Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial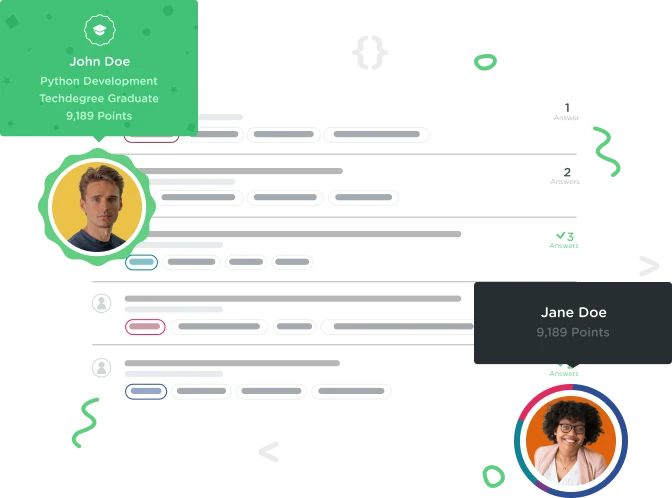

Nicolas Queen
7,331 PointsAny thoughts on my answer to this challenge?
var quizQA = [
["What year did Fry get locked into the cryogenic freezer?", "2000"],
["What job is Zoidberg horrible at?", "doctor"],
["Who is Fry's best friend?", "bender"],
["What is Fry's dog's name?", 'seymour'],
["Who is the most amazing toad ever?", "hypnotoad"]
];
function print(message) {
document.write(message);
}
var guessCorrect = 0;
var guessIncorrect = 0;
var answer;
for (var i = 0; i < quizQA.length; i++ ) {
answer = prompt(quizQA[i][0]);
if ( answer.toLowerCase() === quizQA[i][1]) {
guessCorrect += 1;
print("<p>Correct! You got <strong>'" + quizQA[i][0] + " </strong> right!</p>")
} else {
guessIncorrect += 1;
print("<p>Sorry! You got <strong>'" + quizQA[i][0] + " '</strong> wrong!</p>")
}
}
if ( guessCorrect >= 3) {
print("<h1>Great job! You got '" + guessCorrect + " right and " + guessIncorrect + " questions wrong.</h1>")
} else {
print("<h1>On no! You got " + guessCorrect + " right and " + guessIncorrect + " wrong. You should go watch more Futurama!</h1>")
}
2 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsSo just a couple of suggestions/comments:
First, you don't really need a variable to store the number of incorrect answers, when you can subtract the number of correct answers from the length of the array of questions.
Second, you could change the last conditional to do a bit of simple math to determine the message to display instead of using a fixed number, so if you add more questions, it still makes sense. Perhaps greater than the length of the questions array divided by 2 (i.e. more than half right)?
That way, if you added more awesome Futurama quiz questions, the user would still need to get a decent amount right to be properly praised! :)

Nicolas Queen
7,331 PointsThanks. I changed it a bit after the second part of the challenge and put it on codePen for those curious :
Chris Shearon
Courses Plus Student 17,330 PointsChris Shearon
Courses Plus Student 17,330 PointsAll hail hypnotoad!!
I haven't tried it out but I like your use of a 2d array.