Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial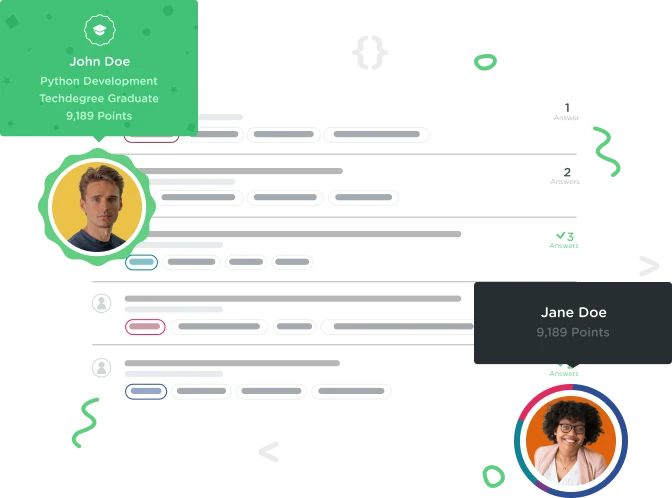

Adesh chavan
Courses Plus Student 35 PointsAnyone Suggest Me ruby method to count consecutive number of 1's
Sample Case 1: The binary representation of 5 is 101 , so the maximum number of consecutive 1's is 1 .
1 Answer
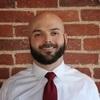
John Baulig
9,180 Pointsdef consecutive(number)
number = number.split('') # turns number string to an array
array = [] # empty array to hold all counts of consecutive ones
number.chunk { |n| # chunk method from enumerable module
n == '1' # chunk is filtering for 'chunks' of '1' and 'chunks' of 'not 1'
# example: an array of [0, 1, 1, 2, 3, 5, 1, 9] will return
# [false, [0]] [true, [1, 1]] [false, [2, 3, 5]] [true, [1]] [false, [9]]
}.each do |x| # we want to loop through each of the above arrays
if x[0] == true # we want to separate the chunks of ones which are 'true' in index position 0
array.push(x[1].count) # filling the empty array with counts of ones in each chunk of ones
end
end
array.sort! # sorts the counts in ascending order | from the above example: [1, 2]
puts array[-1] # puts the last number in the array as that would be the greatest #
end
John Baulig
9,180 PointsJohn Baulig
9,180 PointsHope this helps!
Let me know if you have any questions.