Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial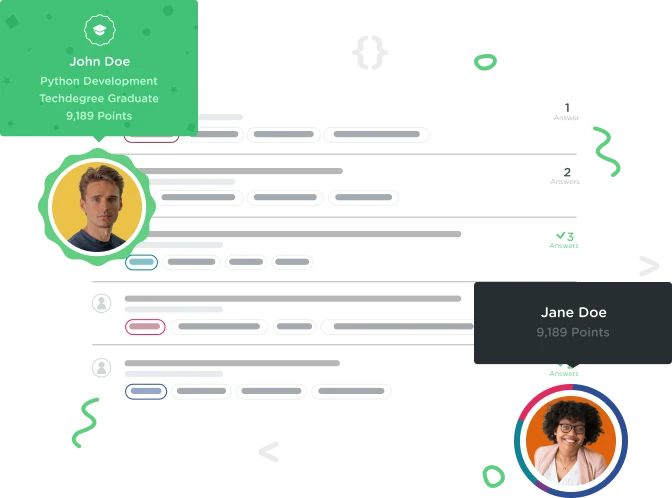
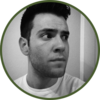
Stephen Santiago
9,631 PointsArray & Loops Code Challenge - What am I doing wrong?!?!?!
I am supposed to Iterate each value in the Array "temperatures". Not sure what I am doing wrong exactly.
var temperatures = [100,90,99,80,70,65,30,10];
function temp(temperatures) {
for ( var i = 0, i < temperatures.length, i += 1 ) {
console.log(temperatures[i]);
}
}
temp(temperatures);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
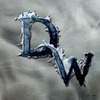
Hugo Paz
15,622 PointsHi Stephen,
You have commas instead of semi colons in your for definition.
for ( var i = 0, i < temperatures.length, i += 1 )
should be
for ( var i = 0; i < temperatures.length; i += 1 )
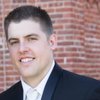
Austin Lilley
5,727 PointsI think this should do it for you:
var temperatures = [100,90,99,80,70,65,30,10];
function temp(temperatures) {
for (var i = 0; i < temperatures.length; i ++ ) {
console.log(temperatures[i]);
}
}
temp(temperatures);
My changes:
- changed the"," in your for loop to ";"
- updated "i += 1" to "i++"
Let me know if that doesn't solve your issue.
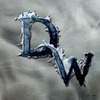
Hugo Paz
15,622 PointsHi Austinlilley,
removing the var is a really bad idea. Javascript will assume its a global variable and if you have the a variable with the same name somewhere else in your code, it can cause problems.
By declaring it inside the function with the keyword var, you ensure that its scope is limited to the function so if you have a global variable called i it will not be affected.
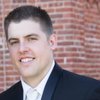
Austin Lilley
5,727 PointsMy mistake. I removed that bit of advice, thanks for catching that :)
Stephen Santiago
9,631 PointsStephen Santiago
9,631 Points-_- I'm an idiot, thank you