Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial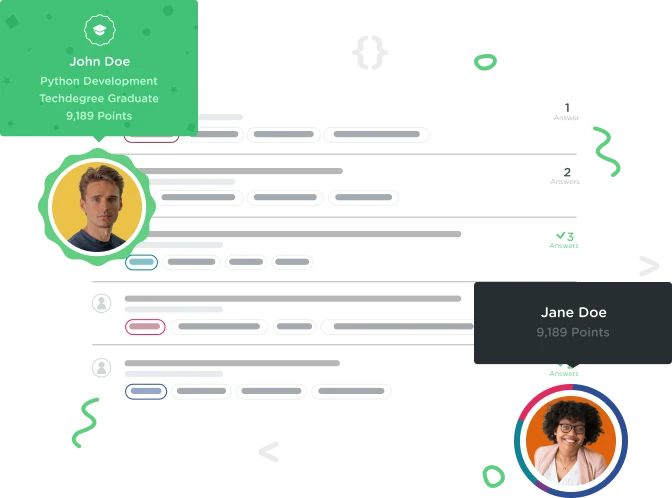

Anthony Branze
4,887 PointsArray of answers?
I am just starting my challenge.. and as I am going through the requirements, I am struck by this question.. While asking our 5 questions. Should we have a list of the correct answers to check user input against? or am I just over thinking a very simple step and just use prompt and check against the answer directly in if?? say
var questionOne = prompt("is yes or no");
if (questionOne === "yes") {
correctAnswers++;
}
2 Answers
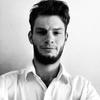
Rich Turnbull
9,212 PointsTaking it further, you could use an array of objects to store your questions and answers:
//Variables
var score = 0;
var questions = [
{
text: "What is the abbreviation of Kentucky?",
answer: "KY"
},
{
text: "Is cheese delicious?",
answer: "NO"
},
{
text: "What type of animal has spots and a long neck?",
answer: "GIRAFFE"
}
];
//code to run
for(i=0;i<questions.length;i++){
var current_question = questions[i];
var guess = prompt(current_question.text);
if(guess.toUpperCase() == current_question.answer){
score += 1;
}
}
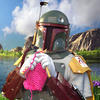
Lindsay Sauer
12,029 PointsHi Anthony,
I'm not familiar with what the challenge requires, but from a functional standpoint you could go about it as you have written, by checking if the user's answer is equal to a specific value.
Another way to do it would be like you were thinking and have an array of answers, with each index in the questions array matching the index in the answers array:
var questions = ["What is the abbreviation of Kentucky?", "Is cheese delicious?", "What type of animal has spots and a long neck?"];
var answers = ["KY", "Yes", "Giraffe"];
var correctAnswers = 0;
for (var i=0; i<questions.length; i++) {
var userAnswer = prompt(questions[i]);
if (userAnswer === answers[i]) {
correctAnswers++;
}
}
Lindsay Sauer
12,029 PointsLindsay Sauer
12,029 PointsRich's answer is much cleaner and complete, even if he's a cheese-hating heretic!
Anthony Branze
4,887 PointsAnthony Branze
4,887 PointsSee that's where I was originally going in my head but then I started second guessing if I actually could do it that way then I started thinking this is supposed to be "beginner" and using objects doesn't come until later. I'm really good at doing the opposite of what we are supposed to do when breaking down problems into smaller problems I tend to go to far or over complicate things... This for example.
But rich your answer is exactly where my mind was going.