Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial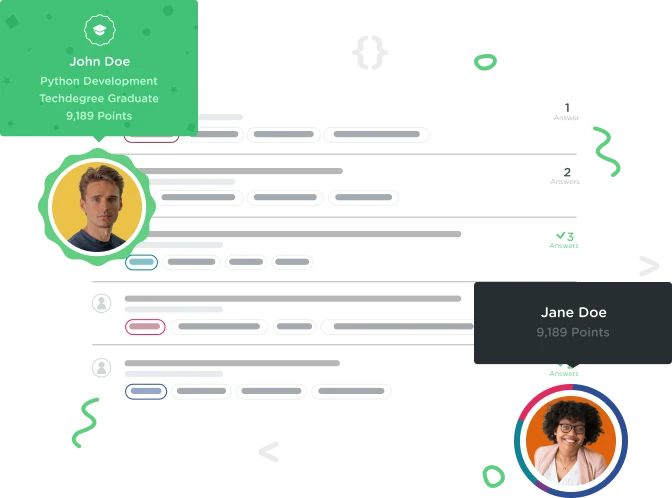

dlpuxdzztg
8,243 PointsArrayList in SQLite
I'm trying to create a SQLiteHelper for my 'ListItem' object. This is my code so far:
private static final String DB_NAME = "easylist.db";
private static final int DB_VERSION = 1;
// List table functionality...
public static final String LISTS_TABLE = "LISTS";
public static final String LISTS_NAME = "NAME";
public static final int LISTS_CHECKED = 0;
public ListSQLiteHelper(Context context) {
super(context, DB_NAME, null, DB_VERSION);
}
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
}
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
}
However, my ListItem object also has an ArrayList value, which I also intent to save:
private ArrayList<TaskItem> mListsList; // The list's array of tasks
private String mListName; // The name of the list
private int mImportant; // Whether the item is important
Edit: My TaskItem objects have values of their own:
private String mTaskName; // Name of task
private int mChecked; // Whether the item is checked
How would I save an ArrayList in an SQLiteHelper object?
1 Answer

Ben Deitch
Treehouse TeacherI'd probably store the tasks array as a delimited String, and then recreate the tasks when you load the data. For example, if I have to take out the trash and mow the lawn, then I'd use this String (using a tilde ~ for the delimiter): "take out the trash~mow the lawn".
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsHow would I go about saving the other values in a delimited string?
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherWhat other values do you have?
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsShoot, I should have mentioned this earlier; each TaskItem object in my list has their own string and int values. I'll post the code for the TaskItem values.
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherYou just need an extra delimiter: "take out the trash$$$0~mow the lawn$$$1"
Here's some code that shows how to do it (it's in Kotlin but it should be very similar for Java):
Prints:
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsInteresting, I might actually look into that first before proceeding; this might not be the only case I need this.