Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial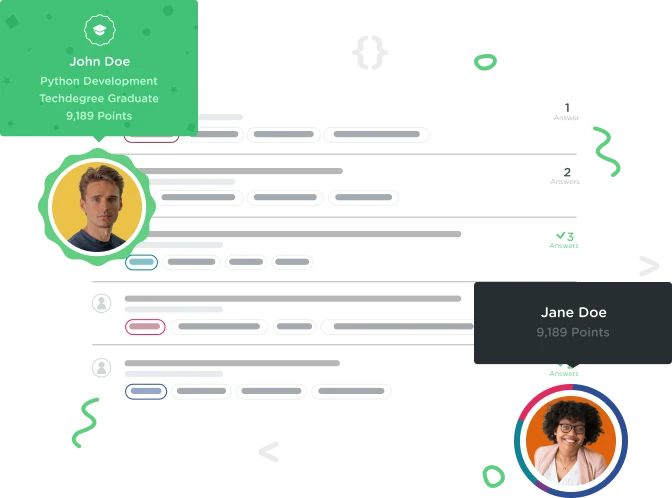
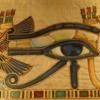
Gregory James
6,537 PointsAttributeError tuple 'upper'
The videos weren't helpful in explaining the .remove. I am having trouble understanding a function. I was able to understand it just fine with Ruby but with Python, I don't understand how to properly set up a function. Yes, I looked back at the functions video but I can't see to being myself to just make a functioning "function". I am also getting a tuple error which I don't understand why. Nothing that I search is really explained.
def disemvowel(word):
vowels = "a","e","i","o", "u"
vowels = vowels.upper()
vowels = vowels.lower()
try:
word.remove(vowels)
except ValueError:
pass
return word
1 Answer

turaboy holmirzaev
19,198 Points- The input to your function is a type of String and String does not have "remove" method but "list" does.
if you use remove on a string it will throw an error.
You can use "remove" method on a list. It will remove the item but if you try to remove item not in the list, it will throw an error(value error)
lists are mutable that's why try not to make any changes to your list while it is being worked on. I will show you what I mean.
understand iterables. If something can be iterated over, you can call them as iterables. So "string", tuple, list ...are examples of iterables.
tuples do not have "upper" or "lower" methods. These methods belong to "string"
# Strings
word="someword"
#word is string now. So you can do
word = word.upper()
# now word is "SOMEWORD"
# and if you assign it again, it is gonna change
word = word.lower()
#now word is "someword"
#if you try remove method on word now it will throw an error
#in your case, you will need to change string to a list
#example
word = "someword"
wordlist = list(word) #this will generate ["s", "o", "m", "e", "w", "o", "r", "d"]
#you can also make a string from list by using "join" method
word = "".join(wordlist) #join method makes string out list "someword"
#Lists
worldlist = ["s", "o", "m", "e", "w", "o", "r", "d"]
#you can remove items from the list
wordlist.remove('o') #remove changes your list permanantly so worldlist now ["s", "m", "e", "w", "o", "r", "d"]
# 'in' operator checks if something is a member
#>>> "a" in "someword" returns false
#>>> "s" in "someword" returns true
# you will make use of "in" operator in your task
# Your challenge task is:
#make a string using vowel letters vowels = "aeoui"
#make a list from the input word. wordlist = list(words)
#iterate over input word using for loop
# inside for loop check if letter is in vowels string
# if letter in vowels string, remove the letter from wordlist (nor from word)
# once the loop is done
# make a new string from wordlist using "join" method and return that new string
#to start
def disemvowel(word):
vowels = "aioue"
wordlist = list(word)
#for loop here
#example
myword = "treehouse"
for letter in myword:
print(letter.lower())
for letter in myword:
if letter in "challenge":
print(letter)
Gregory James
6,537 PointsGregory James
6,537 PointsI feel like i am close but I still don't quite understand why it is not working.
def disemvowel(word): vowel= "aeiou" wordlist= list(word) myword= "Ebony" for letter in myword: print(myword) if letter in myword: myword.remove(wordlist) return word = "".join(wordlist)