Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial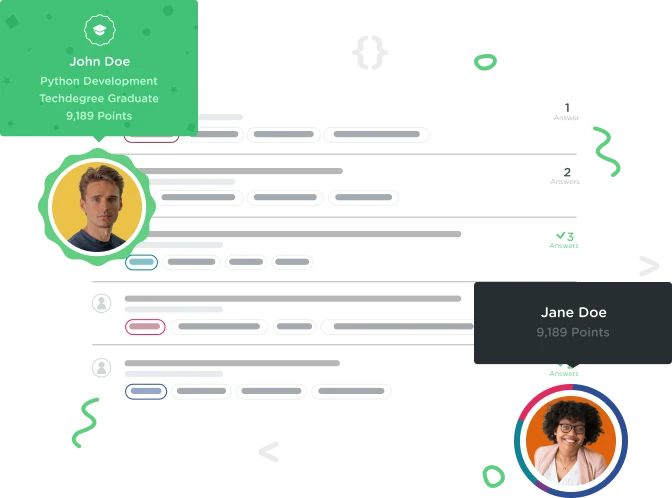

dlpuxdzztg
8,243 PointsBest Method for Saving Checkbox Items (and whether they're checked or not) in a RecyclerView
Hello.
I wanted to know what was the best way to save a list of RecyclerView items that contain a checkbox, including whether it is checked or not.
Thank you.
2 Answers
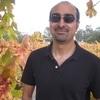
Kourosh Raeen
23,733 PointsThere are a few options for persisting data. You could use a SQLite database or Shared Preferences. Using a database is a lot more work but sometimes that's the right solution. Shared Preferences is usually used for persisting settings as key-value pairs. The values can be primitive data types, not custom objects which is what you have, but you can use the Gson library to get around that limitation. Take a look at this page for more information:
https://guides.codepath.com/android/Persisting-Data-to-the-Device
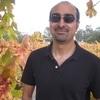
Kourosh Raeen
23,733 PointsMaybe you can use a HashMap<Integer, Boolean> in the adapter to store the position of the CheckBox in the list and its state. Using a click listener, every time a CheckBox is clicked you will add its position and state as a key-value pair to the HashMap. Then in onBindViewHolder
you can use the position to look up the state of the CheckBox and set it accordingly.

dlpuxdzztg
8,243 PointsWhat if I already have an ArrayList in my adapter? Should the ArrayList and the HashMap be two different entities?
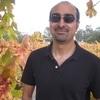
Kourosh Raeen
23,733 PointsWhat data is the ArrayList holding?

dlpuxdzztg
8,243 PointsThe ArrayList is holding a custom object (ListItem in my case.)
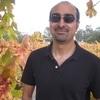
Kourosh Raeen
23,733 PointsI'm not quite sure how to use that ArrayList to keep track of the state of the CheckBox, unless the custom object you're holding in the ArrayList has a property for the state of the CheckBox, like a boolean field. The reason I suggested a HashMap is because you'll need to hold two pieces of information for each row in the list, one is the position within the list and the other is the state of the CheckBox.

dlpuxdzztg
8,243 PointsI was actually thinking about implementing a boolean property in my custom object when creating it, I think I'll do just that, and maybe change the Array to a HashMap.
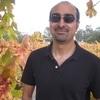
Kourosh Raeen
23,733 PointsIf you're going to add a boolean property then I think you won't need the HashMap anymore. You can just listen for changes to the state of the CheckBox in the ViewHolder and when a change happens you set the boolean property on the custom object accordingly. Then in onBindViewHolder
you get the boolean value form the object and use it to set the state of the CheckBox.

dlpuxdzztg
8,243 PointsAh, okay. But what about saving the data in the list? What would be the best method for saving the data in my list?
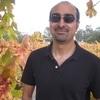
Kourosh Raeen
23,733 PointsIs this data entered by users? I assumed the ArrayList you were referring to already contained some custom objects and it was passed to the adapter by the activity/fragment.

dlpuxdzztg
8,243 PointsYes, the data is entered by users.
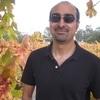
Kourosh Raeen
23,733 PointsI see. And are you using the data entered by the user to create instances of ListItem
? Can you tell me how the user interacts with the list? From a previous question you'd asked, I remember that each row of the list contains an ImageView and a CheckBox. I'm just wondering what the data entered by the user is, where it was entered, and how it corresponds to the rows/items in the list.

dlpuxdzztg
8,243 PointsThe user enters the data in a DialogFragment using an EditText, the data from the EditText is then displayed as the text used for the CheckBox. The ImageView is a button that allows you to remove the item.
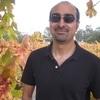
Kourosh Raeen
23,733 PointsIs there a field in the custom object for storing the data entered into the EditText? If there is then you can set it at the same time that you set the boolean field with the state of the CheckBox. Are you looking for a way of persisting this data, like in a database or something?

dlpuxdzztg
8,243 PointsMy object has a Boolean field (for checkbox state) and a String field (for checkbox name). Oh yeah, persisting is the word I'm looking for, I'm just not sure where or how to do it.