Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial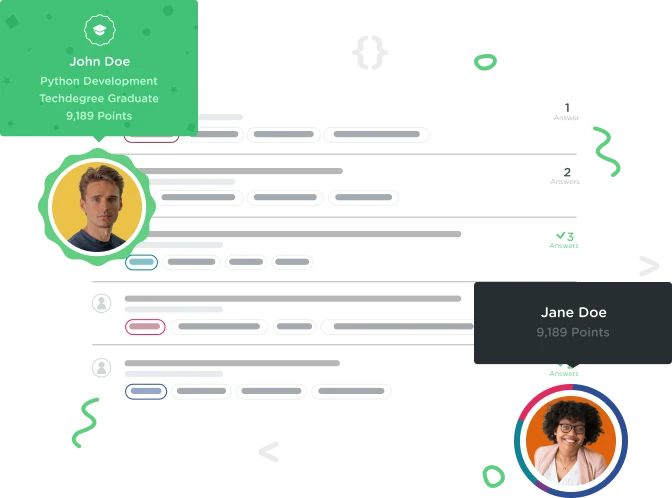

dlpuxdzztg
8,243 PointsCalling a DialogFragment from a RecyclerView
Hello.
I wanted to know if there was a way I could call a DialogFragment from a RecyclerView. Let's say I want to press something in my ViewHolder that would open up a DialogFragment, how would I do that?
Thank you.
2 Answers
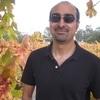
Kourosh Raeen
23,733 PointsHi Diego - You can create a custom click listener interface in your RecyclerView adapter and then have your activity/fragment containing the RecyclerView implement this interface. The interface will have one method that you implement in the activity/fragment and in the body of that method you create and show your custom DialogFragment. You then call this method in your ViewHolder class in the adapter. Take a look at the code in this link for more detail:
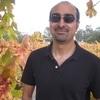
Kourosh Raeen
23,733 PointsCan you post the body of the onItemClicked()
method? Is that defined in an activity/fragment? I assume that's where you're creating an instance of PromptDeleteDialogFragment
, is that correct?

dlpuxdzztg
8,243 PointsThis is everything I have for my onItemClicked() body (MainActivity):
@Override
public void onItemClicked(View v) {
}
The thing is that the button I want to press is in the ViewHolder for my RecyclerView, should I implement the listener there?
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsOkay. But what if I want to press a button in my DialogFragment, how would I access the button I want to press if the fragment is it's own class?
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsIn the
onCreateView
method of the class that's extending DialogFragment you can get a reference to the button usingfindViewById
and then set a click listener on it.dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsI meant from my ViewHolder; I want something to be pressed in my ViewHolder that opens up the DialogFragment. I was curious because I'm still having trouble accessing my DialogFragment in my ViewHolder.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsIf you have a reference, in your ViewHolder, to the item that you want to press that opens the DialogFragment then you should be able to call
setOnClickListener
on it and then in the overriddenonClick
method you can either create the DialogFragment or if you're using a custom click listener interface, as in my post above, you can call the interface's method. If you still have trouble with this please share the code so I can take a look at it.dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsHere is my code for my DialogFragment I want to access:
Code for ViewHolder in RecyclerView (I want the DialogFragment to open when mDeleteItemImage is pressed)
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsWhere are
mListener
andonItemClicked()
defined? Can you post the code for those?dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsSure thing
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsHi Diego - The code to create an instance of your DialogFragment and to show it needs to go in
onItemClicked()
in your MainActivity. I also suggest simplifying the related code in your ViewHolder. Remove the lines:and change the line:
mDeleteItemImage.setOnClickListener(listener);
to:
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsAlright, it's somewhat working; I'm getting an error when I press my button.
I believe it has something to do with the creation of my DialogFragment.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsThe error might have to to with when you're calling
create()
onbuilder
. See if moving this line:AlertDialog dialog = builder.create();
to right before the return statement would get rid of the error.
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsI got it working! But what if I want to call dialog.setContentView()? How would I do that?
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsWhy do you need to call
setContentView()
? Haven't you already inflated the layout? By the way, I'm not sure if you've seen this tutorial so I'll post the link here. You may find some useful tips here: https://guides.codepath.com/android/Using-DialogFragment