Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial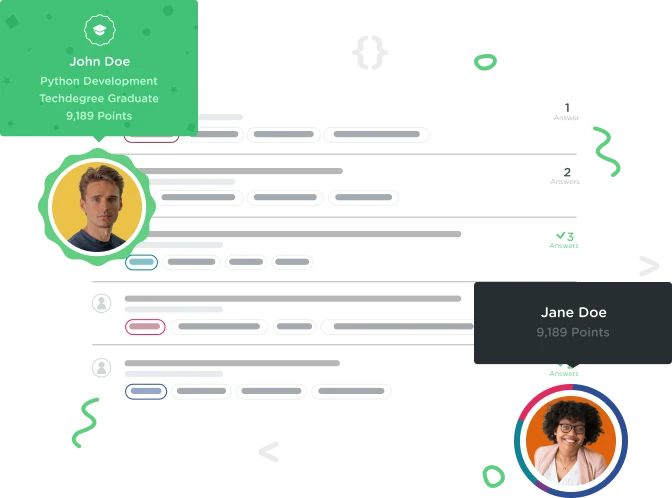

Md Akibe Hashan
2,422 Pointscan anyone please explain me how every line is working in this code
e
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_dict = {}
word_list = string.split(" ")
for sel_word in word_list:
count = 0
for word in word_list:
if sel_word == word:
count +=1
word_dict[sel_word] = count
return word_dict
1 Answer

James Joseph
4,885 Pointsstring = string.lower() - You've now convered the arg you parsed in the function to all be lower case word_dict = {} - You've created a dictionary called word_dict word_list = string.split(" ") - You've now created a list based on the lower cased string split (FYI using " " isn't needed to split the line from spaces :) you also could've done this in one line)
string = string.lower().split()
Now lets get into the for loop you've done
for sel_word in word_list:
count = 0
for word in word_list:
if sel_word == word:
count +=1
word_dict[sel_word] = count
for sel_word in word_list - For each item in word_list count = 0 - You're setting up a default value of count
Nested for loop for word in word_list - For each item in word_list if sel_word == word - If the first item in for loop is equal to the nested item in that for loop count +=1 - Increase the counter by 1 word_dict[sel_word] = count - Append dictionary with key word sel_word and add the value of count
What this for loop and nested for loop is doing is this: Lets say the word_list is this: ['i', 'do', 'not', 'like', 'it', 'sam', 'i', 'am']
The first loop is going to grab the first item "i" The nested for loop is then going to go through all of the values in word_list and check if if there are any values that match the letter "i" if it does it will increase the counter by 1 It will then add the value of "i" as a key and add the value of counter into the dictionary.
It will then start the process again with "do", "not" etc
It's actually a very nice way of doing it :)
However, a quicker and less lined way of doing it is:
for sel_word in word_list:
word_dict[sel_word] = word_list.count(word)
return dictionary
Count is a function that lists have that allows you to count the amount of times the value exists in the list, you can then simply add the value as the key and the count value as the dictionary value.
Hope that helps.