Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial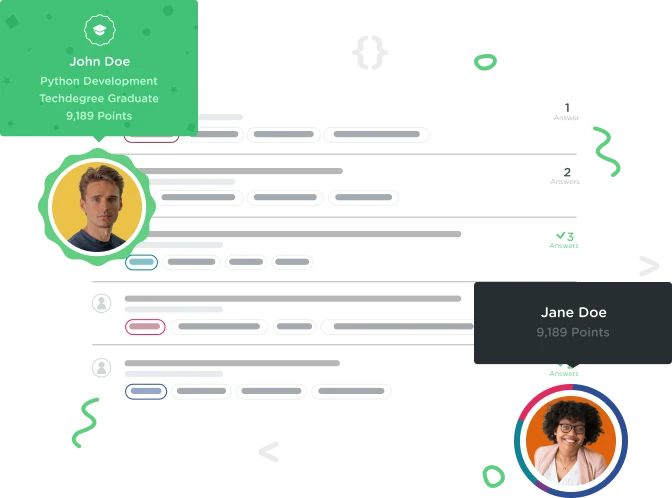

Carey Cooke
3,952 PointsCan someone explain this to me please?
I had some trouble with the last search challenge but after reading through some questions and answers I got it to work. However, I have a question on the found variable and hoping someone can explain it to me and why it's set the way it is.
var message = '';
var student;
var search;
var found = false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: Type a name or quit to end');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
if (search != "") { // Why is this set to an empty string?
found = false; // particularly this, I don't get why it's set to False and what it's being used for?
for (var i = 0; i < students.length; i += 1) { // this I understand
student = students[i]; // this I understand
if (student.name.toLowerCase() === search.toLowerCase()) {
message += getStudentReport( student ); // this I understand
print(message);
found = true; // back to this found. Not sure why a user was using it and what the purpose is?
}
if (!found) { // Why isn't this set to something? I know ! means not, so if we set found = false is that the same thing?
print("<p>Student named " + search + " not found</p>");
}
}
}
}
2 Answers

Jeff Lemay
14,268 Pointswhile (true) {
search = prompt('Search student records: Type a name or quit to end');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
if (search != "") { // If the search query is empty, we don't need to test anything. Someone just hit the Search button without entering a keyword in the search field
found = false; // We just want to confirm that we start with a false value. If matches are found, we'll set it to true later on
for (var i = 0; i < students.length; i += 1) { // this I understand
student = students[i]; // this I understand
if (student.name.toLowerCase() === search.toLowerCase()) {
message += getStudentReport( student ); // this I understand
print(message);
found = true; // If the search came back with matching results, we set our found value to true which is used in a later if statement to determine whether to show the "no matches found" response
}
if (!found) { // If found is true (isset), we want to show the results. If found is not set (this if statement would validate as true), we want to tell the user that their search term was not found
print("<p>Student named " + search + " not found</p>");
}
}
}
}

Iain Simmons
Treehouse Moderator 32,305 PointsIt's very important to understand that the code within the parentheses for the if
statements are conditionals, and aren't assigning a value to a variable, they are comparing it to something, including the last one, where it's comparing 'not found
' to the boolean value of true
.
So yes, as you asked, !found
is the same as found === false
.
If you don't like the use of the boolean, you could instead check if message
still equals an empty string:
if (message === '') {
print("<p>Student named " + search + " not found</p>");
}