Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial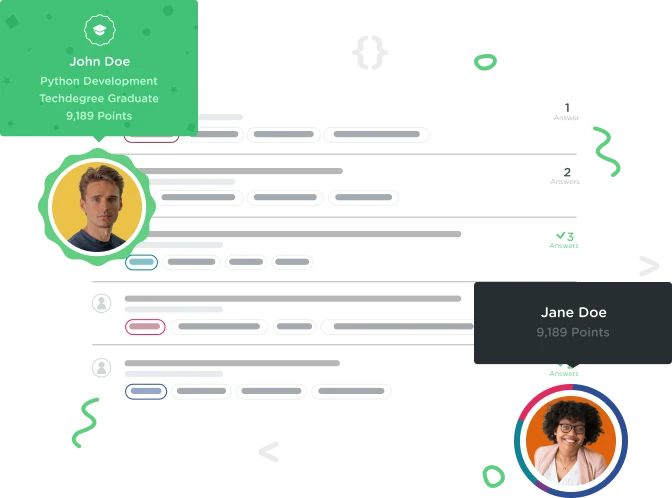

romankozak2
5,782 PointsCan someone explain why the print() function is not inside the loop? I tried it that way and it printed each item twice.
Can someone explain why the print() function is not inside the loop? I tried it that way and it printed each item twice.
I assumed that since it is looping through, it would add the information to the message then print that object, followed by the next object, and the next object. Why does it print the object properties more than one when print(message) is inside the loop? Just looking for some logical clarity here.
Thank you!
1 Answer
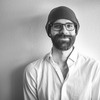
Joseph Fraley
Treehouse Guest TeacherGreat question Roman. I was a little confused looking at the code at first myself.
I think your idea is that the loop builds up a message for each student. And so you expect it to print the message out when it's done, for each student. That's a great intuition, and a totally valid way to solve this problem.
However, what's actually happening in the instructor's code is different. Let me put here for reference:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for ( var i = 0; i < students.length; i++ ) {
student = students[i];
{ a bunch of code regarding the student }
}
print( message );
So why doesn't this print(message)
call happen inside the loop, so that the message for each student gets printed to the page as the loop iterates? Maybe like this:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for ( var i = 0; i < students.length; i++ ) {
student = students[i];
{ a bunch of code regarding the student }
print( message );
}
Well, that produces really weird results. Some students information seems to get printed more than once. Yikes! The code is structured such that the message
variable is defined globally, before the loop starts running. The function print(message)
doesn't print an individual student's info to the page, although we could re-write it to do so. Rather, the loop builds up the message
string so that it contains ALL of the student's info, and then we print()
the WHOLE collection to the page at one time.
There are some reasons to prefer this approach to the solution you expected. If we build up the whole collection of students, and then print the whole set in one action, rather than printing them one at a time as we complete them, the browser doesn't have to make as many calculations about its appearance. Thus, there is a marginal performance improvement for putting everything on the screen all at once, instead of one student at a time. (we sometimes call the browser's changes to its own appearance a "repainting" event).
Other than that, nothing prevents us from writing this function to be more like the one you expected:
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for ( var i = 0; i < students.length; i++ ) {
var message = '';
var student = students[i];
{ a bunch of code regarding the student }
print( message );
}
Now the message
and student
variables are re-assigned each time the loop runs, and we call print( message ) at the end of each loop. Thus, the window will get a new student string for each iteration of the loop, rather than one big one with all the students at once.
Does that answer your question? If so, please up-vote my answer as "Best Answer"! It helps me reach my career path goals quickly. If not, feel free to write more!
Ahmed Alhaj
Courses Plus Student 35 PointsAhmed Alhaj
Courses Plus Student 35 PointsAll data from students array were stored in the variable message then we simply printed it out the loop.