Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial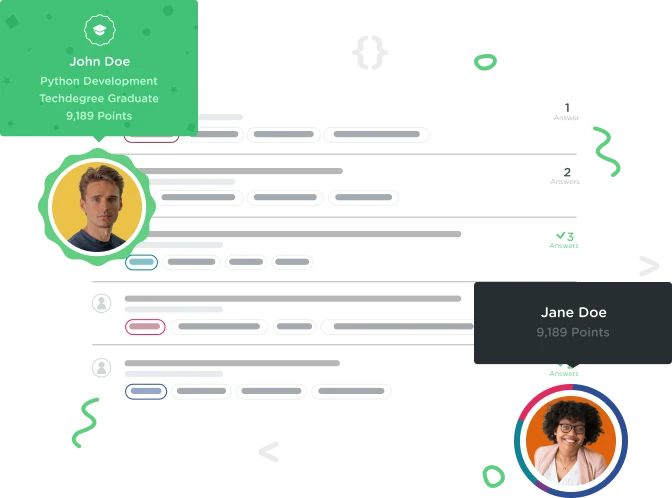

Marguerite Holden
6,075 PointsCan someone help me here. I've gotten thinngs right and still got error messages so I don't know if this is right or wro
It's the else statement I'm unsure of here.
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return safe
else
return unsafe
end
3 Answers
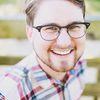
Jacob Herrington
15,835 PointsHey Marguerite,
Ruby is a weird (but great) language -- and you are experiencing some of its weirdness. There are a couple of syntax issues in your code, but don't worry, syntax errors happen to every developer no matter how "good".
Read my comments in this snippet:
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return safe #The variable safe is undefined here
else
return unsafe #The variable unsafe is undefined here
end
#We are missing something here
So let's figure this out, line by line:
# Here is our starting code
# Notice that the function (code under the def line) has an "end" all to itself, this lets Ruby know that
# the function is over and it should move to the next block of code.
def check_speed(car_speed)
# write your code here
end
Lets add our If/Else statement to figure out if the vehicle is moving quick enough -- your logic was 100% correct here, so I won't explain that too much.
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50 #IF condition THEN
# do something
else #IF not condition THEN
# do something
end #An "end" just for the if statement
end #We keep our end for the function down here!
Now the final step is to return something. The challenge asks for strings, so let's make sure that is the data type we use.
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return "safe" #We have to return "safe" not safe. The first one is referring to a string, the second a variable.
else
return "unsafe" #We have to return "unsafe" not unsafe. The first one is referring to a string, the second a variable.
end
end
So the only problem you had there was confusing how Ruby sees strings -- a string in Ruby has to be surrounded by 'single quotes' or "double quotes", otherwise Ruby thinks you are talking about a variable name.
So the final passing code would be:
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return "safe"
else
return "unsafe"
end
end
Let me know if I can make this more clear!!
edit: thanks Jason for pointing out that indentation is not important in Ruby.
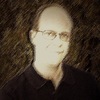
Jason Anders
Treehouse Moderator 145,860 PointsHey Marguerite,
Your really close. There are just 2 things:
- When returning "safe" and "unsafe", they need to be strings. Right now, as it is, the compiler is looking for variables named
safe
andunsafe
. So, just change those to strings. - There is an
end
missing for the if clause.
Good Job & Keep Coding! :)
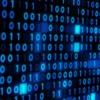
Alexander Davison
65,469 PointsLol our answers are nearly the same XD
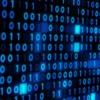
Alexander Davison
65,469 PointsBy the way, how did you make "safe" and "unsafe" into that monospace font?
Just curious :)

Jason Anello
Courses Plus Student 94,610 PointsHi Alexander,
Inline code can be wrapped with single backticks.
Here's more details on posting code: https://teamtreehouse.com/forum/posting-code-to-the-forum

Marguerite Holden
6,075 PointsThanks for explaining how Ruby sees variables from strings. I think I've made this error before which confuses me when looking at error messages.

Marguerite Holden
6,075 PointsThanks for the clarity here between variables and strings.
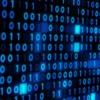
Alexander Davison
65,469 PointsYou did great! Here's the things that are causing the errors:
- You forgot to put double/single quotes around the "safe" and "unsafe" strings.
- You also forgot to put an "end" for the check_speed function.
If you fix those, your complete code should look like this:
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return "safe"
else
return "unsafe"
end
end
Good luck! ~alex
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Jacob,
Indentation doesn't matter in ruby. It would matter in python, for example.
The end keyword determines where the code belongs.
Jacob Herrington
15,835 PointsJacob Herrington
15,835 PointsHaha, nice catch Jason Anello -- I'm much more versed in Python!!