Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial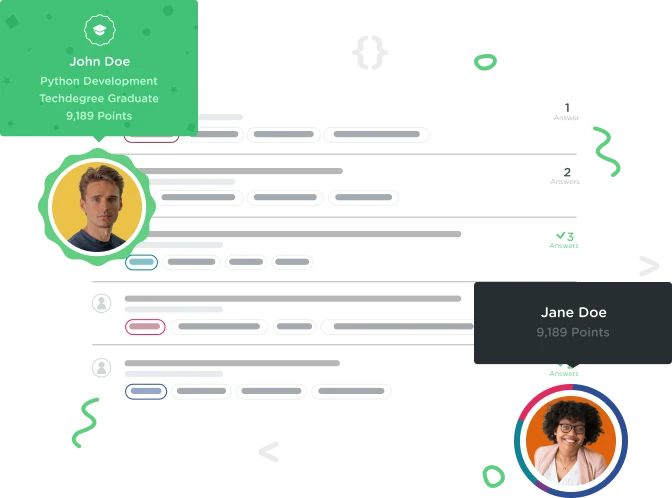
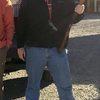
Alec Chiarotti
2,025 PointsCan someone please show me this completed I am extremely confused, thank you!
Both task 1 and 2. I've tried for hours and I'm pretty lost.
2 Answers

ve
5,048 PointsThe first task is: Create a while loop. This while loop shall run, while the value of your counter is smaller than the number of ints inside your array "numbers". You can check for the number of items inside an array using the .count-method on it. Don't forget to increment your counter each time the while-loop runs, or else you'll create an endless loop!
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count{
counter+=1
}
Task two: Everytime the loop runs, you add the next number inside your array to the variable sum. Remember: You access the values inside an array using the index value. So numbers[0] is 1, numbers[1] is 8 and so on. Good you have your variable counter: The first time your loop runs, your variable counter has the value of 0 and increments each time your loop runs! So you can use counter to access the items inside numbers:
while counter < numbers.count{
sum+=numbers[counter]
counter+=1
}
Dan Lindsay
39,611 PointsHey Alec,
So for the first part, we need to see up our while loop, like so:
while counter < numbers.count {
counter += 1
}
This is saying that while our counter variable is less than the number of items in our number array, we are going to increment our counter variable by 1.
For the second part of the challenge, we need to draw out each item in our numbers array, and add them to our sum variable. Remember, to access individual elements of an array, we can use the index of the array. If we wanted the first number we would say:
numbers[0]
if we wanted the third number we would use:
numbers[2]
and so onβ¦ The challenge asked us to use the counter variable as the index value, so weβll create a new variable to store the value before we add it to our sum, like so:
while counter < numbers.count {
var newValue = numbers[counter]
sum += newValue
counter += 1
}
This will pass the challenge.
The first time through the loop, counter will be 0, the first number from our numbers array will be added to sum and then the counter will increment by one. The second time through, the counter is now 1, so weβll get the next number in our numbers array and add it to sum, and our counter is now incremented up by 1, making it 2 now. This process will keep going until our counter is less than the total count of our numbers array(numbers.count).
It should be noted, that you can pass the first part of the challenge with the following code:
while counter > numbers.count {
counter += 1
}
but when you get to part 2, adding any code to our loop will never run, as our counter will never be greater than numbers.count and our counter will never be incremented. You may have done everything else right, but used > instead of <.
Hope this helps you out!
Dan
Dan Lindsay
39,611 PointsIt looks like Valerie must have already answered you while I was typing all this out. Can never tell if someone has answered while you're typing in this comment field.
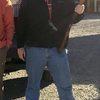
Alec Chiarotti
2,025 PointsThank you, Dan!
Alec Chiarotti
2,025 PointsAlec Chiarotti
2,025 PointsWow, thank you so much for not only breaking it down for me and showing me how to solve it, but the for quick response as well!