Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial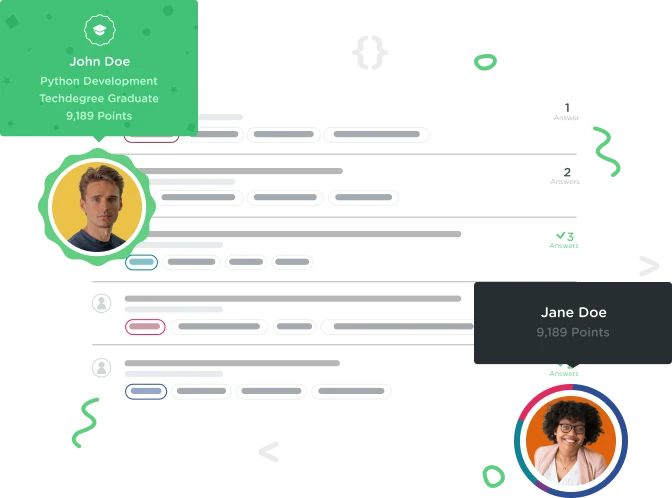

khabir baukman
436 Pointscan someone tell me what i have wrong in this program
i think i have everything correct, but i'm not sure what the question means when it says return none.
def add(a, b):
try:
except ValueError:
return None
else:
c = float(a)+float(b)
return c
add(1, 2)
1 Answer
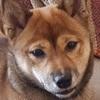
Katie Wood
19,141 PointsHi there,
The issue seems to be how the try-except is formatted. Your 'try', 'except', and 'else' should all line up. The challenge also asks that your 'try' block include the code to convert the values to floats. This part is important, because the code in the 'try' block should be the code that could possibly throw an error.
You have a couple of options to solve this - one is to separate the conversion from the addition, and place the return in the 'else' block by itself:
def add(a, b):
try:
float_a = float(a)
float_b = float(b)
except ValueError:
return None
else:
return float_a + float_b
The other way is to just put the whole return statement in the 'try' block:
def add(a, b):
try:
return float(a) + float(b)
except ValueError:
return None
I tried both - both should pass the challenge. Feel free to use whichever one you like better - hope this helps!