Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial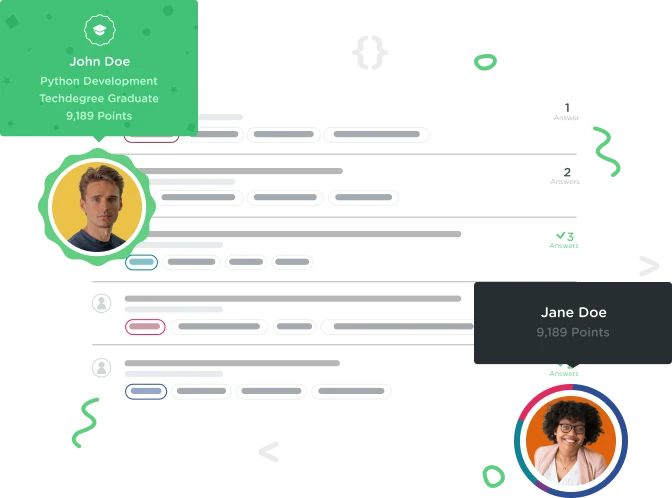

Terry Felder
1,738 Pointscan write a for loop, cant seem to put into a function, does enumerate take argurments?
so i been in my repl.it just working away at this problem. Thought i try first to just put the example enumerate('abc...') into the format they want it, I feel pretty sure this is a good code for it. but because of my lack of understanding in enumerate. im wrecking my brain to wrap it all in a function, was there something i missed? im thinking zip function could help this problem but i dont want to take shortcuts and i want to apply what im learning .... AND if im even approaching this the wrong way i am all open for suggestions im ready to learn.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# def combo(item1,item2):
new_list = []
for index, letter in enumerate('abcdefghijklmnopqrstuvwxyz'):
together = index,letter
new = tuple(together)
new_list.append(new)
print(new_list)

Demetrius lewis
10,424 PointsHey Terry, you have the right idea but your code doesn't include an of the parameters to append to the new list. Make sure that you can appending item1 to the new list matching the index of item2 and then return the new list to complete the challenge. I'll add a helping hand in the answer area below. Hopefully i don't give away to much to make it too easy for you.
3 Answers

Demetrius lewis
10,424 Pointsdef combo(?, ?): new_list = [] for i, item in enumerate(?): new_list.append((?, i?[i])) return new_list
(Fill in the question marks.)

Terry Felder
1,738 Pointsdef combo(item1,item2):
new_list = []
for a, b in enumerate(item1):
together = a,b
new = tuple(together)
new_list.append(new)
return new_list
print(combo('abc',[1,2,3])) ?? right??

Christopher Frederick
6,547 PointsAs you suspected (and as I hinted), this can also be completed with zip in a greatly-simplified manner:
def combo(item1, item2):
together = zip(item1, item2)
print(list(together))
Zip alone returns a zip object and its location in memory, so it is necessary to convert that into a list prior to printing. This could be made even simpler by doing everything inside print:
def combo(item1, item2):
print(list(zip(item1, item2)))
Keep playing with this in the repl and I'm sure you'll get it with enumerate as well. :)

Terry Felder
1,738 Pointslol the second i tried to use zip() , the Error message told me not to use zip....but i think i figured it out...
def combo(item1,item2):
new_list = []
for a, b in enumerate(item1):
together = a,b
new = tuple(together)
new_list.append(new)
return new_list
print(combo('abc',[1,2,3]))

Terry Felder
1,738 Pointsp.s i appreciate your response guys! ive been back and forth reading and going over the work and results trying to fully comprehend what everyone is saying and everything im doing its a really big help guys thank you so much sorry i havent had time to reply but like i said taking your guys code working and playing and re reading your answers is bringing everything together! THANKS AGAIN!
Christopher Frederick
6,547 PointsChristopher Frederick
6,547 PointsHi Terry,
Enumerate takes an iterable and returns it with a counter; by default this starts at 0. What your current code is doing is taking your string and assigning each letter a counter number from 0-25 (since we're not starting at 1; would look like
enumerate('somestringhere', 1)
) .Your variable
together
is actually already a tuple, so there is no need to convert into a tuple and reassign tonew
(remember, you can always check what you're getting by usingtype()
).The primary issue with your code currently is that it doesn't take into account that you need to zip together (if you catch my drift) two different iterables, but as it is right now, you are essentially ignoring the second iterable. There are a bunch of different ways to handle this code challenge, but if you really want to make use of enumerate, consider utilizing the counter to help you access items in your other iterable to pair with the first.