Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial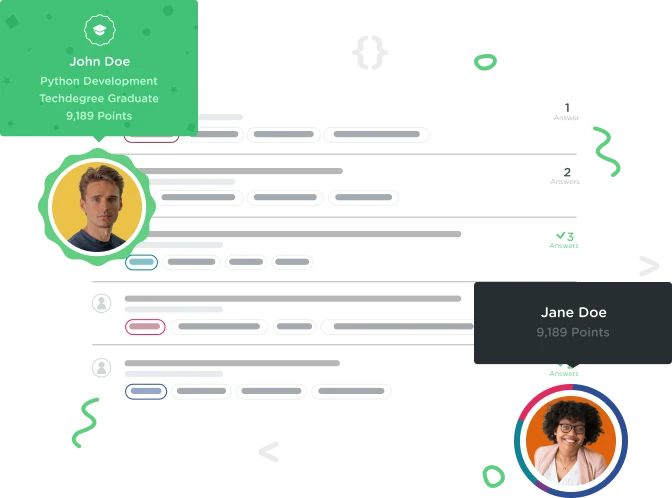

Gary Gibson
5,011 PointsCannot get program to end with phrases for right or wrong answer.
I have copied the code from the lesson (with the addition of one 'quit' at line 36 for my own practice) and I still cannot get the program to end with "You got it" if all the guessed letters are correct or "You didn't get it" if the seven guesses get used up.
import random
words = [
"apple",
"banana",
"orange",
"coconut",
"strawberry",
"lime",
"grapefruit",
"lemon",
"blueberry",
"melon"
]
while True:
start = input("Press any key to start, or enter 'quit' to quit.")
if start.lower() == "quit":
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print("")
print("Strikes: {}/7".format(len(bad_guesses)))
print("")
guess = input("Guess a letter: ").lower()
if guess == 'quit':
break
if len(guess) != 1:
print("You can only guess a single letter a time!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess the word. My secret word was {}.".format(secret_word))
MOD corrected markdown for readability of code.
3 Answers

Gavin Ralston
28,770 PointsWhen you add a letter to the good_guesses variable, you're only adding one. If the letter appears multiple times in a word, though, you'll always have a word length longer than your good_guesses length, which means you'll never be able to win. This is because the win condition is having as many good guesses as there are letters in the secret word.
Try adding this to the code to follow along:
if guess in secret_word:
good_guesses.append(guess)
print("Good guess accepted, I now add one letter to the good_guesses variable.")
print("good_guesses length is now {}".format(len(good_guesses)))
print("secret_word length is {}".format(len(secret_word)))
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
It'll look something like this if the word comes up "Strawberry" and very similar for other words with repeating letters.. like coconut, or blueberry. Orange should work just fine for either condition though.

Gary Gibson
5,011 PointsWhat's funny is my random generator picks "blueberry" the most and "strawberry" second most.
Thank you very much for the help.
Running into these snags and then seeing how they're fixed....really helps me understand better.

Gary Gibson
5,011 PointsOkay, I thought that last option was working and it wasn't.
It also didn't work when I changed "list" to "set" up top.
What DID work was changing "list" to "set" down at the bottom..
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(set(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
I ran the code several times with each change and just changing "list" to "set" in this block was the charm.
You are correct: I have not gotten to sets yet at this part of the course. But I can see how sets work to account for repeating letters here so that my program breaks even with "strawberry" and "blueberry" instead of just with "orange" and "lime" and "melon"!
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsI see what you're saying. Someone else was trying to explain this to me, too. I guess I'm getting confused because the instructor's code worked on the video as I have reproduced it here.
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsWell, ONE way to handle it would be to create a set of the letters in secret word. I'm not sure if you're there yet in the course, but since a set only contains unique items, it'd be sure to have all the letters only once. So this would work:
while len(bad_guesses) < 7 and len(good_guesses) != len(set(secret_word)):
Or down at the bottom (or just set the win count somewhere else and check them both)
Another way of doing it would be to use a for loop to go over each letter and be sure to add a letter in good_guesses each time it shows up. That might make more sense to you at this point since you're introduced to for loops but haven't probably seen sets in action.
I think the problem with the code in these videos is Kenneth Love didn't actually run into any words during these demonstrations that actually included multiple occurrences of a letter. He got, like, "melon" and "lime" and maybe "orange" but he never ran into any berries or coconuts. So he didn't hit this particular snag. His code would have worked just like yours if he tried to correctly guess "blueberry"