Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial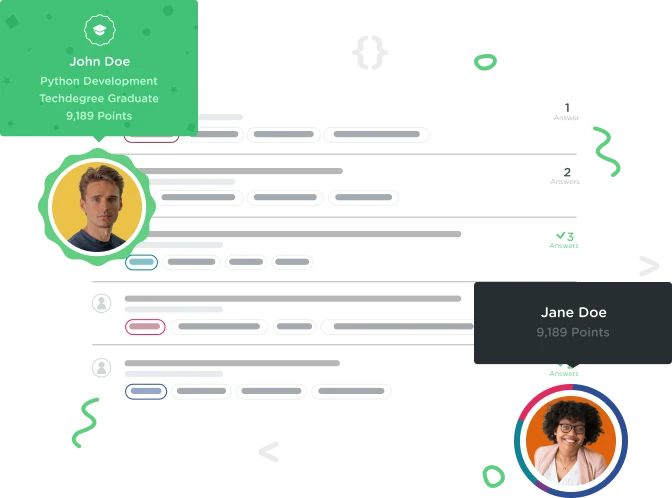
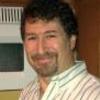
Fred Tarbox
Courses Plus Student 17,110 PointsCannot read property 'Symbol(Symbol.iterator)' of undefined
This code works in Codepen but not here
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers.reduce((a, personal) => { [ ...a, ...personal.hobbies];}, []);
//console.log(hobbies);
1 Answer
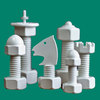
Steven Parker
231,275 PointsI get the exact same error in Codepen, as I would expect.
There's two syntax issues:
- when defining an arrow function with brackets, you must return the result
- "hobbies" is not a direct property of a "customers" array item (which is what "personal" represents)
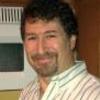
Fred Tarbox
Courses Plus Student 17,110 PointsHere's my exact code in Codepen:
hobbies = customers.reduce(function(prev, curr) { return [ ...prev, ...curr.hobbies]; }, []); console.log(hobbies);
and here's the result:
["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
I put this same code into the Challenge Task and get the error
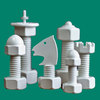
Steven Parker
231,275 PointsThat's a bit different from what you show above, but it only resolves one of the two issues, and still produces the same error instead of that result on Codepen.
Since "hobbies" is not a direct property of a "customers" array item, you need to access it via the "personal" property ("curr
.personal
.hobbies
").
Fred Tarbox
Courses Plus Student 17,110 PointsFred Tarbox
Courses Plus Student 17,110 PointsGot it, thanks!