Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial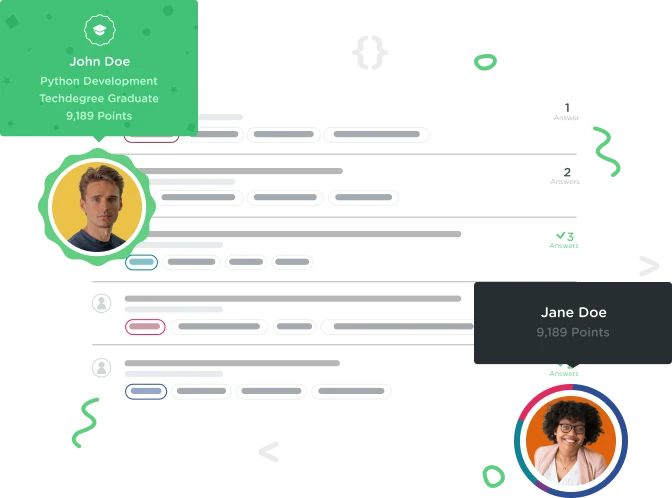

James Gill
Courses Plus Student 34,936 PointsCan't figure out how to iterate dictionary to add values
I'm not sure how to accomplish this task, and I've done a lot of research. I think I'm missing some key clue about iteration on dicts. Please see the comment in the middle. The code challenge is:
"Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value."
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(a_string):
#split the string into a list.
split_string = a_string.split()
#iterate here, doing some gymnastic stuff that assigns a value to each dict item.
return split_string
5 Answers
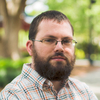
Kenneth Love
Treehouse Guest TeacherJust a comment on your example code. You're not splitting the string into a dictionary. Splitting a string always results in a list.
Then, between for key in list:
and if key in dict:
, you should be able to piece it together.
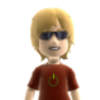
Stephen Goeddel
11,239 Pointstry this:
string_dict = {}
for word in split_string:
if string_dict[word]:
string_dict[word] += 1
else:
string_dict[word] = 1
This should do it, we are just iterating through your list of words in the string and checking to see if each one exists in the dict. If it does we are just incrementing the value stored at that key, and otherwise we are just going to store 1 at that key. I have not ran this code so it may not be perfectly syntactically correct, but I hope that you can get the general idea from it.

James Gill
Courses Plus Student 34,936 PointsSorry, that doesn't work for me. But I see what you're saying.

James Gill
Courses Plus Student 34,936 PointsI solved it! I'd had most of what you suggested, but I was confused about testing for the existence of the word in the dict. So, your line "if string_dict[word]" should be "if word in string_dict", as Kenneth hinted at in the code comments.
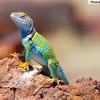
Adam Oliver
8,214 PointsI'm still having problems getting this to work
def word_count(string):
my_dict ={}
x = string.lower().split()
for item in x:
my_dict[item] = 1
if item in my_dict:
my_dict[item] +=1
return my_dict
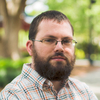
Kenneth Love
Treehouse Guest TeacherLet's look at your code.
def word_count(string):
my_dict ={} # set up a dict
x = string.lower().split() # turn the lowercased string into a list
for item in x: # go through the list, one item at a time
my_dict[item] = 1 # set that item, in the dict, to 1
if item in my_dict: # if whatever `item` is is in the dict
my_dict[item] +=1 # increment its value
return my_dict # return the dict
So when your if
statement runs, it's going to check item
. item
, at that point, will be the last thing in the list. Since it was already put into the dict, it will, indeed, still be in the dict and will increment the value. So every word will end up in your dict w/ a value of 1 and then the last word will get a value of 2.
You need to combine your for
loop and your if
condition so it checks each word to see if it's in the dict. If it is, increment the value. If it's not, set the value to 1.
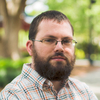
Kenneth Love
Treehouse Guest TeacherThe solution to this is pretty close to the solution to the previous code challenge, Membership.

James Gill
Courses Plus Student 34,936 PointsThanks. Wish I'd saved that code. :]
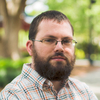
Kenneth Love
Treehouse Guest TeacherJames Gill there are definitely times it would be handy to be able to go back to your solution for a CC. I'll suggest that to our devs.
That said, solving the CC again just further reinforces the processes, right? :)

James Gill
Courses Plus Student 34,936 PointsRight. No pain, no gain. To be honest, the Python collections problems have helped me level up a little in "thinking like a programmer".
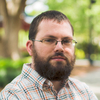
Kenneth Love
Treehouse Guest TeacherJames Gill Object-Oriented Python is coming next month. That should help more, too.
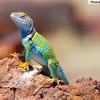
Adam Oliver
8,214 Pointsi had my_dict[item] +=1 and then had the else statement assign 1 to everything else.
Thanks for the help.
James Gill
Courses Plus Student 34,936 PointsJames Gill
Courses Plus Student 34,936 PointsYou're right, I realized that after I'd posted--and from sussing out clues contained in error messages.