Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial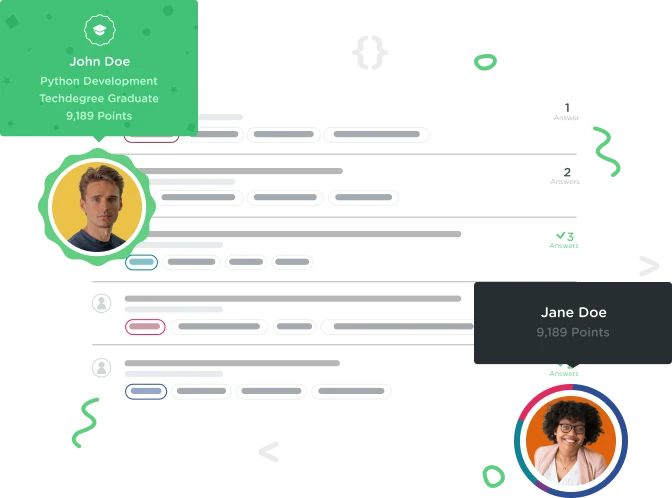

travis ueki
2,102 PointsCan't figure out the data type of lists in order to result in my for loop removing the nested list
In the last elif I want to check the condition of (psuedo code)
for item in list: elif item == list: the_list.remove(item)
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
newItem = the_list.pop(3)
the_list.insert(0, newItem)
def cleanUp(list):
for item in list:
if item == 'a':
list.remove('a')
print(list)
continue
elif item == False:
list.remove(item)
print(the_list)
continue
elif item == type(list):
list.remove(item)
print(list)
continue
cleanUp(the_list)
1 Answer
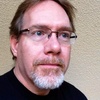
Chris Freeman
Treehouse Moderator 68,441 PointsThe statement you are looking for is isinstance(item, list)
to check if item is of type list
. There are two issues with this approach.
You are using the key word
list
as your argument name forcleanUp
which overwrites the namespace andlist
is no longer thetype
but instead has the value of the argument passed tocleanUp
. It is not a good idea to uselist
or any keyword as a variable name. Is is common to you "lst" for a list variable.You are operating on the same list you are looping over. What happens is the list is shortened by the
remove
actions then the loop thinks it's done since the length is less and doesn't loop over the last item.
also, since all the code covered by an if
or elif
, the continue
statements aren't needed:
newItem = the_list.pop(3)
the_list.insert(0, newItem)
def cleanUp(mylist):
for idx, item in enumerate(mylist[:]):
if item == 'a':
mylist.remove('a')
elif item == False:
mylist.remove(item)
elif isinstance(item, list):
mylist.remove(list(item))
cleanUp(the_list)
The last item can also be removed using the_list.pop()
which removes the last item in the list:
the_list.remove('a')
the_list.remove(False)
the_list.pop()