Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial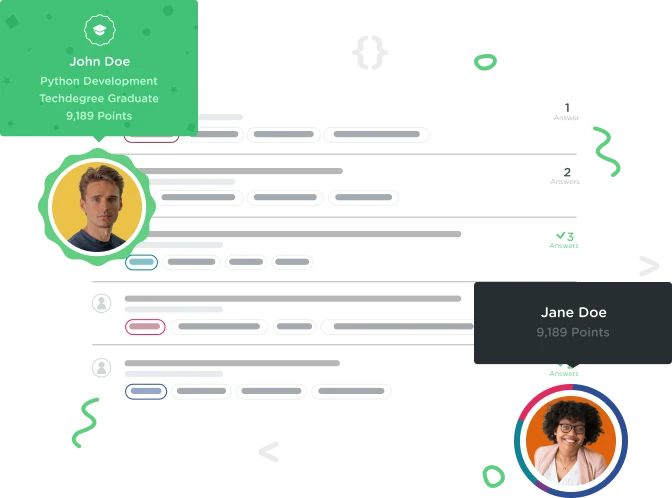
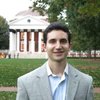
Jonathan Weinstein
9,063 PointsCan't figure out why only the first entry is appearing! Anyone see the error?
I'm trying to get all the students and their data to print out like it is for the first one. Anyone know why nothing else comes after that? Thanks!
var students=[
{name: 'John',
track: 'iOS',
achievements: 7,
points: 654
},
{name: 'Sara',
track: 'Web Design',
achievements: 34,
points: 67
},
{name: 'Orlo',
track: 'Front End Development',
achievements: 35,
points: 1048
},
{name: 'Patrick',
track: 'Ruby',
achievements: 66,
points: 1236
},
{name: 'Jane',
track: 'iOS',
achievements: 45,
points: 6689
}
];
function html() {
for (var i = 0; i < students.length; i +=1) {
var entry = "<h2>Student: " + students[i].name + "</h2>";
entry += "<p>Track: " + students[i].track + "</p>";
entry += "<p>Achievements: " + students[i].achievements + "</p>";
entry += "<p>Points: " + students[i].points + "</p>";
return entry;
}
}
document.write(html(students));
2 Answers

LaVaughn Haynes
12,397 PointsYour return statement is inside the loop so you return entry on the first iteration. You should also define your variable outside of the loop. Finally you you are passing students when you call html() but there is no argument for it in your function.
function html(students) {
var entry = '';
for (var i = 0; i < students.length; i +=1) {
entry += "<h2>Student: " + students[i].name + "</h2>";
entry += "<p>Track: " + students[i].track + "</p>";
entry += "<p>Achievements: " + students[i].achievements + "</p>";
entry += "<p>Points: " + students[i].points + "</p>";
}
return entry;
}

Leonardo Hernandez
13,798 PointsThe problem has to do with your loop and the return in the loop. The reserved word 'return' causes the loop to stop on the the first iteration and you only get John.
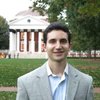
Jonathan Weinstein
9,063 PointsThanks! I moved the return statement to outside the loop, but, as I just noted above, now I get only the last entry instead of the first...
Leonardo Hernandez
13,798 PointsLeonardo Hernandez
13,798 PointsJohnathan doesn't need to pass the parameter though right? The object 'students' is in scope. To make a function that prints object property values (on any object) the 'for in' loop could be a solution that would require a parameter.
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsYou are correct. In this situation he does not need to pass students in. But if he wanted to have multiple student arrays (var thirdGrade = [{}], fourthGrade[{}];) it would make his function more reusable to pass it in and not use the global. Also if he decides not to pass in students then he could just call the function like this
document.write(html());
Jonathan Weinstein
9,063 PointsJonathan Weinstein
9,063 PointsHi LaVaughn, I added in your solution, but now instead of getting only the first entry, I get only the last entry! It seems like var entry is being rewritten each time it goes through the loop, not simply added to like what we want. Any thoughts on this?
LaVaughn Haynes
12,397 PointsLaVaughn Haynes
12,397 PointsSee the example I posted above. It's because you defined the variable inside of the loop like this:
var entry = "<h2>Student: " + students[i].name + "</h2>";
move the var entry part outside of the loop and just concatenate the string inside the loop like this:
you are erasing the contents of your var on each iteration
Jonathan Weinstein
9,063 PointsJonathan Weinstein
9,063 Pointsspot on