Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial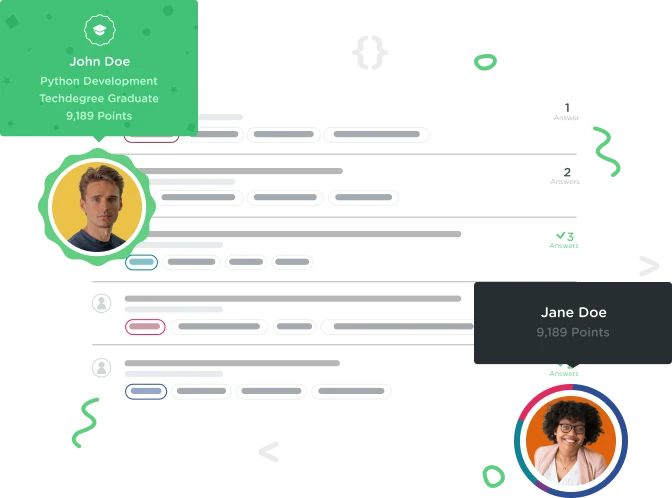

Michael Zito
Courses Plus Student 4,073 PointsCertainly not correct, but am I close?
I feel like I'm getting there, but need a walk through.
function max (7, 11) {
return 7, 11;
if {
11 >= 7;
}
else {
7 >= 11;
}
}
1 Answer

Joseph Wasden
20,406 PointsOne nudge I'd give is to name the arguments in your function declaration; don't put actiual number values you intend to use with the function yet.
For example:
function max (num1, num2) {
return num1, num2
if {
num2 >= num1
}
else {
num1 >= num2;
}
}
There are other enhancements needed, of course. something to consider; can you return comma separated values? Also, what will happen to the code I declare under my return statement? will it ever run?
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/return

Michael Zito
Courses Plus Student 4,073 PointsThank you! very helpful
Jamie Carter
Front End Web Development Techdegree Student 12,096 PointsJamie Carter
Front End Web Development Techdegree Student 12,096 Pointsnot far off.
Firstly your function arguments are slightly off. Currently you are passing 7 and 11 in as arguments. But to be more universal you should name these.
an if statement always needs a condition, then an action
else does not need a condition, it will occur if the condition is false.
My final answer would be:
To run said function and pass in real numbers:
max(10,100)