Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial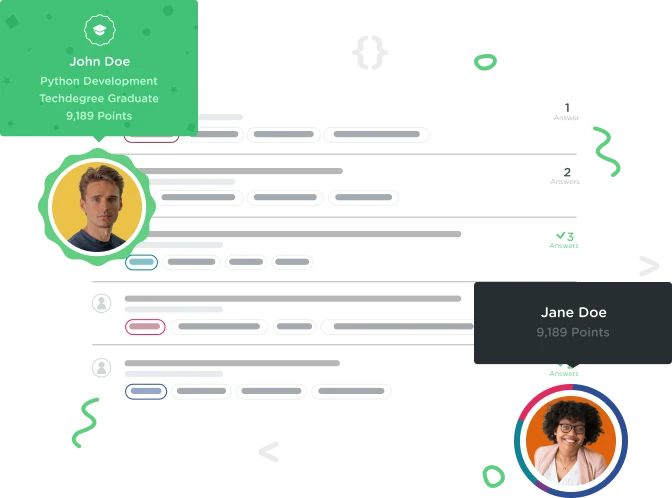

guarlonmirka
10,192 PointsChallenge completed, I need feedback please.
I have completed my challenge. Is there an expert in the field who look at it and give me his feedback?
//The Conditional Challenge
alert("If you are ready for a challenge? then just answer the following 5 questions to win a Gold Crown");
var Q1 = prompt("What is the capital of France?"); /*Ask a question*/
var A1= Q1.toUpperCase();/*Convert the answer given by the user to an Uppercase and assigne a new variable to it*/
alert('Your answer is ' + A1);
var correctAnswer1 = "PARIS";/*Assign a variable to the correct answer*/
//alert('The correct answer is ' + correctAnswer1);
/*Use a conditional statement and a boolean, if the user answered correctly (True) the user get 1 point */
if (A1===correctAnswer1){
var scoreQ1 = 1
// alert('your score is '+ scoreQ1);
}else{
var scoreQ1 = 0
// alert('your score is '+ scoreQ1);
}
var Q2 = prompt("What is the capital of Algeria?");
var A2= Q2.toUpperCase();
alert('Your answer is ' + A2);
var correctAnswer2 = "ALGIERS";
//alert('The correct answer is ' + correctAnswer2);
/*Use a conditional statement and a boolean, if the user answered correctly (True) the user get 1 point */
if (A2===correctAnswer2){
var scoreQ2 = 1
// alert('your score is '+ scoreQ2);
}else{
var scoreQ2 = 0
// alert('your score is '+ scoreQ2);
}
var Q3 = prompt("What is the capital of Portugal?");
var A3= Q3.toUpperCase();
alert('Your answer is ' + A3);
var correctAnswer3 = "LISBON";
//alert('The correct answer is ' + correctAnswer3);
/*Use a conditional statement and a boolean, if the user answered correctly (True) the user get 1 point */
if (A3===correctAnswer3){
var scoreQ3 = 1
// alert('your score is '+ scoreQ3);
}else{
var scoreQ3 = 0
// alert('your score is '+ scoreQ3);
}
var Q4 = prompt("What is the capital of Spain?");
var A4= Q4.toUpperCase();
alert('Your answer is ' + A4);
var correctAnswer4 = "MADRID";
//alert('The correct answer is ' + correctAnswer4);
/*Use a conditional statement and a boolean, if the user answered correctly (True) the user get 1 point */
if (A4===correctAnswer4){
var scoreQ4 = 1
// alert('your score is '+ scoreQ4);
}else{
var scoreQ4 = 0
// alert('your score is '+ scoreQ4);
}
var Q5 = prompt("What is the capital of Ireland?");
var A5= Q5.toUpperCase();
alert('Your answer is ' + A5);
var correctAnswer5 = "DUBLIN";
//alert('The correct answer is ' + correctAnswer5);
/*Use a conditional statement and a boolean, if the user answered correctly (True) the user get 1 point */
if (A5===correctAnswer5){
var scoreQ5 = 1
// alert('your score is '+ scoreQ5);
}else{
var scoreQ5 = 0
// alert('your score is '+ scoreQ5);
}
/*Assign a user a totalscore */
var totalScore = scoreQ1 + scoreQ2 + scoreQ3 + scoreQ4 + scoreQ5;
alert('your total score is '+ totalScore);
/*Assign a user a crown bassed on his score*/
if (totalScore === 0){
alert('Unfortunently, you scored 0. You did not win a Crown, Try again!');
} else if (totalScore === 1){
alert('You scored 1. You won a Bronz Crown, Try again!');
} else if (totalScore === 2){
alert('You scored 2. You won a Bronz Crown, Try again!');
} else if (totalScore === 3){
alert('You scored 3. You won a Silver Crown, Try again!');
}else if (totalScore === 4){
alert('You scored 4. You won a Silver Crown, Try again!');
}else if (totalScore === 5){
alert('Yes you did it. You scored 5. You won a GOLD Crown!');
};
2 Answers
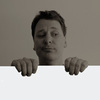
Sean T. Unwin
28,690 PointsNot too shabby. Good job.
A couple of critiques may be:
- to declare at the beginning all of the variables you know you are going to use. JavaScript does this when the script is run, but I mention to do this is because it is easier to read, gives a little clearer picture of the script and also allows you, as the developer, to edit your code later (like when you get into learning about functions for example).
- Unless you are tracking which questions the user get correct or not, I don't think you need to have a separate variable for each question's answer. You could have one variable to track the score and if the question is correct add a point to the score.

guarlonmirka
10,192 PointsThanks guys for you responses, following this advice the programme will be less lengthy and easier to understand. Thanks Rodd for the example :-)
Rodd Castle
29,612 PointsRodd Castle
29,612 PointsI agree with Sean, you could have one variable to track the score and then add to it if the answer is correct: