Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial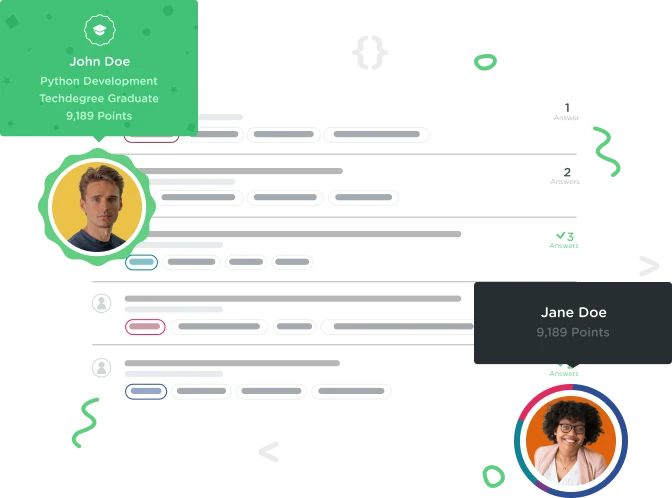

Pau Mayorga Delgado
1,174 PointsChallenge dictionary
The exercise goes like this: "Create a function named most_courses that takes our good ol' teacher dictionary. most_courses should return the name of the teacher with the most courses. You might need to hold onto some sort of max count variable."
The function is the last one, but for some reason it doesn't seem to work. Any ideas?
Thanks!
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(argument):
teachers = len(argument)
return teachers
def num_courses(arg):
num = []
for i in arg:
num_1 = len(arg[i])
num.append(num_1)
total = sum(num)
return total
def courses(argument):
All_courses = []
for i in argument:
n_course = argument[i]
All_courses.extend(n_course)
return All_courses
def most_courses(dic):
max1 = []
for item in dic.items():
a = [item[0]] * len(item[1:])
max1.extend(a)
max_courses = max(max1, key=max1.count)
return max_courses
1 Answer

Kent Åsvang
18,823 PointsI have commented some feedback. I helps if you use print statements to check what is actually happening.
def most_courses(dic):
# Declaring an empty list
max1 = []
# iterating through dict.items()
# dict.items() returns tuples of each entry in a dictionary
# e.g: ("Andrew Chalkley", ['jQuery Basics', 'Node.js Basics'])
for item in dic.items():
# declaring new list 'a'
# item[0] = 'Andrew Chalkley'
# item[1:] = (['jQuery Basics', 'Node.js Basics'],)
# item[1:] is a tuple populated with _one_ item, and its length will always be 1
# [item[0]] * len(item[1:]) is equal to:
# e.g: ['Andrew Clalkley'] * 1 (this will always be one)
a = [item[0]] * len(item[1:])
# extending you list means appending all the items from the iterable you pass
# in as an argument.
# Which basically means that you append every name into this list, multiplied by 1
max1.extend(a)
# list().count is actually a callable method, not an attribute.
# which means you need to call it with an argument.
# e.g: max1.count(argument)
# it will return the number of times 'argument' comes up in the list
# you are using max() here, which might be a neat idea, but this implementation
# doesn't work.
# list().count returns a built-in method, which I believe you are supposed to call
# with an argument. e.g [1, 1, 2, 3].count(1) will return 2. since 1 is represented 2 times
# [1, 1, 2, 3].count however just returns the location in memory,
# I am not sure how that will pan out within the pythons max() function.
max_courses = max(max1, key=max1.count)
return max_courses
This is how I would do it:
def most_courses(dic):
max_count = 0
most_courses = ""
for teacher, courses in dic.items():
if len(courses) > max_count[1]:
max_count = len(courses)
most_courses = teacher
return most_courses
Now, there is a ton of different ways to make this. The trick is to not overthink it. For me it helps to write som algorithmic pseudocode first, before starting on the actual code.
Anyway, hope this helped.
Pau Mayorga Delgado
1,174 PointsPau Mayorga Delgado
1,174 PointsThanks for the comments step by step, it really clarified some things. The part "max(max1, key=max1.count)" i actually got it looking one of the tips in stack overflow, but I have to say that currently I don't think I can use it by my own, it feels too advanced yet. Your method is quite clever, but it worked taking out the [1] from your max_count, otherwise it gave me an error. Thanks again! :)