Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial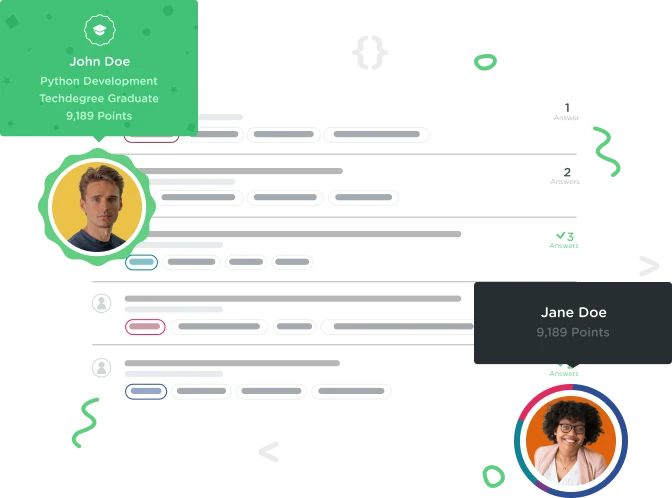

amit dilawri
1,244 PointsChallenge Task 1 of 1 - Using "for" or "while" loop in an array.
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
var temperatures = [100,90,99,80,70,65,30,10];
I used the compare function from Mozilla Developer Network. Solution works on console however this answer was not correct. Any help solving this challenge is much appreciated.
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i += 1){
temperatures.sort(function(a,b) {
return b - a;
})
console.log(temperatures[i] + "<br />");
}
var temperatures = [100,90,99,80,70,65,30,10];
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
5 Answers
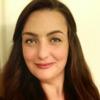
Jennifer Nordell
Treehouse TeacherHi there! The biggest problem here, is that you've gone WAY above and beyond the call of duty here. You've sorted the array. They didn't ask for that so when you log out the numbers they're in the incorrect order. They also do not need you to log out a <br />
. Take a look:
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}
Our temperatures array is given to us by Treehouse. They want us to go through the array and log out the numbers one by one without sorting them first. So we set up our for loop and go through each element and log it out to the console. Hope this helps!

Sun-Li Beatteay
10,606 PointsI think you're just overthinking it a bit. I think they just want a simple for while loop with the console.log method
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i += 1){
console.log(temperatures[i]);
}

amit dilawri
1,244 PointsThanks Jennifer and Sun-Li for your help. It works now. I did spend hours figuring out how to sort the list in descending order.
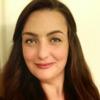
Jennifer Nordell
Treehouse TeacherBut as a bonus, you now know how to sort arrays! And likely as not, you'll need that skill at some point

amit dilawri
1,244 PointsI agree with that.

Chris Drew
5,919 PointsFirst thing is to set up your for loop. how many items are in the array? You could just count the numbers and say something like i < 7; but that code would break if we added any more temperatures.
Instead use the array's .length property. and use i ++ to iterate to the next item in the array each time the code runs.
Since we need to log an array, our console.log statement needs to contain braces.
var temperatures = [100,90,99,80,70,65,30,10];
for (var i=0; i<temperatures.length; i++) {
console.log(temperatures[i]);
}
David Ryan
Courses Plus Student 14,981 PointsDavid Ryan
Courses Plus Student 14,981 PointsI used: +=
This works as well, why?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherDavid Ryan Actually, the code you wrote there will produce a syntax error, but I believe you meant
i += 1
. The codei++
does exactly the same thing asi += 1
. It takes the value ofi
and increments it by 1. The++
operator is used in multiple languages besides JavaScript including(but not limited to), C/C++/C#, earlier versions of Swift, and Java.The benefit to using the
+=
over the++
is that you can specify how much you want to increment by each time. The++
always increments by one. But you could do something likei += 5
, and every time through the loop, thati
variable would increase by 5.Hope this clarifies things!