Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial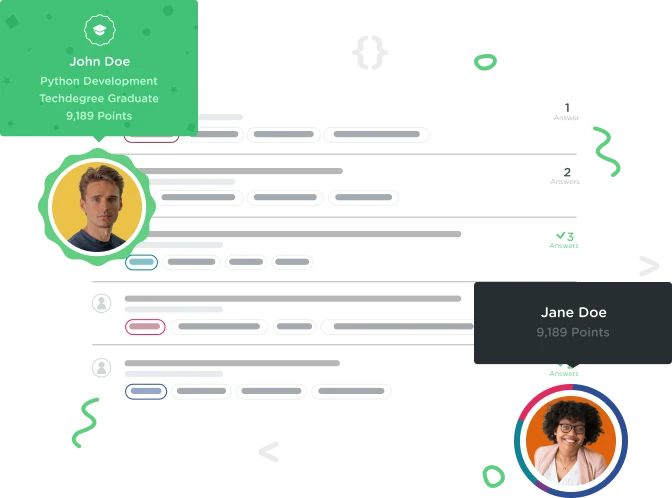

Mike Hill
4,623 PointsChallenge task 3 of 4 of Teachers Stats
Hi there,
I've been working around with this challenge for some time trying to figure out what i did wrong, but still haven't figured it out.
The challenge asks us to "Create a function named stats that takes a dictionary of teachers and returns a list of lists in the format [<name>, <number of classes>]
after entering in my code I get back the response: Bummer! Didn't get the expected output. Got [['Dave McFarland', '1'], ['Andrew Chalkley', '4'], ['Pasan Premaratne', '4'], ['Jason Seifer', '7'], ['Kenneth Love', '2']]
This confuses me because in the dict that i have in my code, most of these teachers aren't in it. I have run my code through an external interpreter and it comes out just fine. I would appreciate any and all help you are willing to give.
~Cheers
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(dict):
maxNum = 0
name = ''
for k,v in dict.items():
if len(v) > maxNum:
name = k
maxNum = len(v)
return name
def num_teachers(dict):
teachers = 0
for k in dict.keys():
teachers += 1
return teachers
def stats(dict):
newList = []
for k,v in dict.items():
newString = '{},{}'.format(k, int(len(v))).split(',')
newList.append(newString)
return newList
8 Answers

Janusz Pachołek
3,334 PointsHi Mike,
Task 3 asks you to "create a function named stats that takes a dictionary of teachers and returns a list of lists in the format [name, number of classes]. " Your code in fact returns a list o lists in format ['string']. In other words, function is expected to return list of lists like this: ['string', integer], your returns: ['string, integer']. I hope I made that clear :) Your code could look like this:
def stats(dict):
newList = []
for k,v in dict.items():
newString = [k, int(len(v))]
newList.append(newString)
return newList
Greetings!
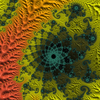
Wes Chumley
6,385 PointsThis one took some serious working through. First Task was tough but eventually deciphered it with some help from the forums and felt it was making sense. Second task was a breeze and the third one just stumped me. Finally got it working well in the workspace interpreter and then when I copied of=ver the code to the task window it gave me an error on TASK ONE!
had to take the whole thing back to the interpreter for debugging and finally sorted it out after modeling Task One after Task Three.
Anyway:
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(classes):
teacher = ()
count = 0
high_count = 0
high_teacher = ()
for name, value in classes.items():
new_teacher = name
count = len(value)
if count > high_count:
high_count = count
teacher = new_teacher
else:
high_count = high_count
teacher = teacher
return teacher
def num_teachers(teachers):
teacher_count = 0
for name in teachers:
teacher_count += 1
return teacher_count
def stats(teachers):
num_classes = []
for name, value in teachers.items():
new_list = [name, len(value)]
num_classes.append(new_list)
return num_classes
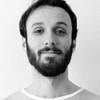
Giorgio Baldelli
6,692 Pointsthanks for your solution Wes!
for name, value in teachers.items():
i'm having some trouble grasping the loop in your solution, in particular the items() method. could you help? thanks!
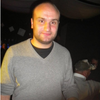
Vittorio Somaschini
33,371 PointsHello Mike.
The challenge does not want strings here; we need the compiler to output a list of lists.
I had a quick look at your code, and apart from the newString line it looks pretty close to a good one.
You already have the teacher, 'k' in your for loop and the classes: 'v'. I would suggest to use the len() method on this value and you would be good to go!
Make sure we get a list for every teacher though!
And let me know if you need more help.
PS: also note that dict is a keyword in python, it is better is you do not use it in cases like this).
Vittorio

Mike Hill
4,623 PointsThank you for your input, i changed the name of the dictionary and will keep that in mind for the future :D

Emmet Lowry
10,196 Pointshey could you explain the 3 challenges a bit more finding it hard to understand what is going on.
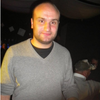
Vittorio Somaschini
33,371 PointsHello Emmet.
What are you finding hard?
The for loop in Janusz code?

Emmet Lowry
10,196 PointsThe for loop gets me all the time and enumeration (dont understand it at all) any help would be great
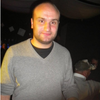
Vittorio Somaschini
33,371 PointsLet's have a look at the for loop:
for teacher, courses in dictionary_one.items():
The handy method items() gives us the chance to basically access all that is inside the dictionary.
This would provide us with the teacher (the key) and the courses (the values).
This is very helpful in our case as we would then need to use both these values for the purposes of the code challenge.
What do you mean by enumeration in this case?
Vittorio

Emmet Lowry
10,196 PointsHi I meant to ask how enumeration works in general not in the example . Thanks

Benedictt Vasquez
2,130 PointsTry this:
def stats(my_dict):
y =[]
for x in my_dict:
z = []
z.append('{}'.format(x))
z.append(len(my_dict[x]))
y.append(z)
return y

Gary Hussain
5,021 PointsThis was challenging but finally got it..
def most_classes(my_dict): maximum = 0 teacher_name = "" for key in my_dict: if len(my_dict[key]) > maximum: maximum = len(my_dict[key]) teacher_name = key return teacher_name
def num_teachers(my_dict): return len(my_dict)
def stats(my_dict): list2 =[] for key, value in my_dict.items(): list1 = [key, len(my_dict[key])] list2.append(list1) return list2
Mike Hill
4,623 PointsMike Hill
4,623 Pointsthank you very much :)