Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial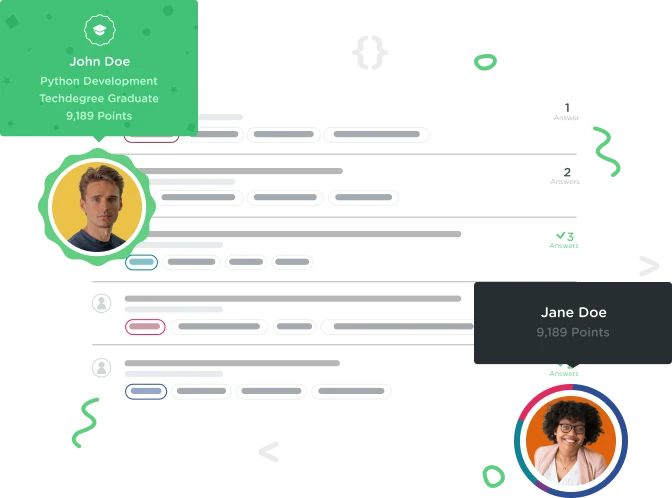

Saman Bir
Courses Plus Student 724 PointsChallenge Task 3 - Try and Exceptions
Hello,
Can someone help me with this?
I have problems starting from "try".
def add(a1, a2):
print(float(a1) + float(a2))
return(float(a1) + float(a2))
try:
a1 = float(a1)
a2 = float(a2)
except ValueError:
return None
else:
return (float(a1)+float(a2))
4 Answers

taejooncho
Courses Plus Student 3,923 PointsSorry about my answer being confusing. Let me clearify step by step. this is your code
def add(a1, a2):
print(float(a1) + float(a2))
return(float(a1) + float(a2))
# This was where I was telling you that was causing the issue
# if you write these two codes before try function and say a1 and a2 is like a list
# python will give you type error before running the command below.
try: <---------# notice how from try and below your code is not indented?
a1 = float(a1) # you want to indent all the code to include into function
a2 = float(a2)
except ValueError:
return None
else:
return (float(a1)+float(a2))
# this line was what I was saying on the side note
# you can write return a1 + a2 and it will give you same results
Your code should look like this to have no error and finish the task
def add(a1, a2):
try:
a1 = float(a1)
a2 = float(a2)
except ValueError:
return None
else:
return a1 + a2
hope this was more helpful. Sorry for not being clear on the first time

Saman Bir
Courses Plus Student 724 PointsHello Taejooncho,
Thank you for your answer. What I really have trouble with is reading the code correctly. For instance this is how I [read] it:
def add(a1, a2):
try:
a1 = float(a1) [a1 should be a float(a1)]
a2 = float(a2) [a2 should be a float(a2)]
except ValueError:
return none [If its not a float don't print any result]
else:
return a1 + a2 [If it is a float print a1 + a2]
add(3, 2.2)
When we type - try: a1 = float(a1)
How do you read it?
The above is my latest try for the challenge. I get the error - NameError: name 'add' is not defined
Sorry for typing this way. I do not know how to get the workspace n the comment section.

taejooncho
Courses Plus Student 3,923 PointsHi there,
As to why I think you are not getting pass the challenge is because of this section from your code on line 2 and line 3
print(float(a1) + float(a2))
return(float(a1) + float(a2))
Since you are doing return float(a1) before the try function starts, it is not working.
For example, let's say that a1 was a list like [1, 2, 3]. You can't make a list into a float, so python will give you an type error before even running the try code down below.
On a side note, when you already made a1 = float(a1) and a2 = float(a2) you can just write
else:
a1 + a2
you do not need to rewrite float(a1) + float(a2) again.

Saman Bir
Courses Plus Student 724 PointsThank you for your help.