Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial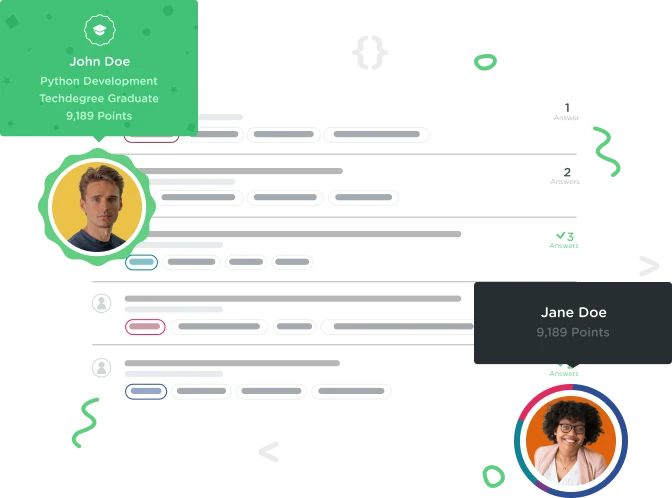

Julie Kohler
6,122 PointsChallenge task, "took too long to evaluate"?
The task was to add a while loop that would keep displaying the prompt until someone answered "sesame".
var secret = prompt("What is the secret password?");
var answer = "sesame"
while (prompt !== answer) {
prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers

Erik Nuber
20,629 Pointsvar secret = prompt("What is the secret password?");
var answer = "sesame"
while (prompt !== answer) {
prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
You are very close. The problem is that prompt is the method you are calling. Secret is the variable you are storing the question into. So when you do your conditional statement in the while loop you should be asking (secret !== answer). Also, when you ask the question in the while loop, nothing but the question is being asked. The answer isn't being stored into anything. So again you need to set secret equal to the prompt.
var userAnswer = prompt("What is the password?");
var secretPassword = 'sesame';
while (userAnswer !== secretPassword) {
userAnswer = prompt("What is the password?");
}
document.write("You know the password. Welcome.");

Julie Kohler
6,122 PointsThanks so much. This clears up a problem I was having with a couple other functions, too. -JAK
Julie Kohler
6,122 PointsJulie Kohler
6,122 PointsOkay, so I have now seen the problem with "prompt" and have now changed it to "secret" in the first part of the while loop instructions. But now the instructions are saying that I need to reassign the value of secret inside the while function. Not sure what that should become. :-/