Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial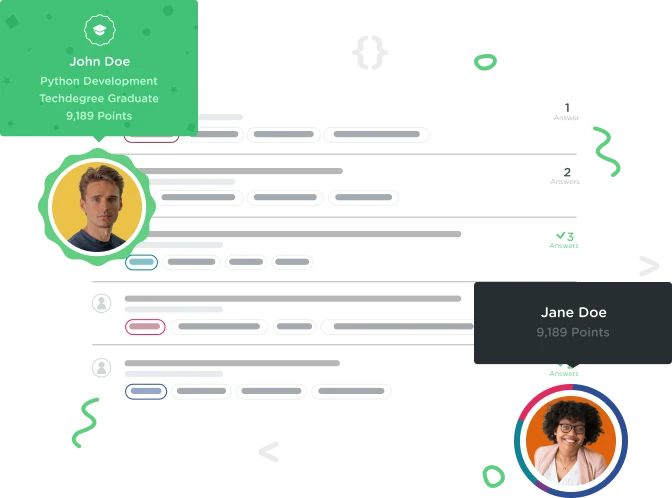
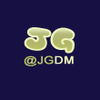
Jonathan Grieve
Treehouse Moderator 91,253 PointsChange default text of a TextView Android
So for practice, I thought I'd make a little app. Click a button change the default text of a TextView component. Nice and straight forward but I can't do it!
I've been able to set up an Activity, with the components I need and the code is free of errors.
Any ideas what I'm missing? I suspect it's a little trickier than I'm trying!
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:id="@+id/RelativeLayout"
tools:context="uk.co.jonniegrieve.newtestapp.TestActivity"
android:textAlignment="center">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Hello World!"
android:layout_alignParentTop="true"
android:layout_marginTop="99dp"
android:textSize="18pt"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:id="@+id/singleText"
android:textAlignment="center"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me!"
android:id="@+id/button"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:background="#fef994"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceLarge"
android:text="Jonnie Grieve Digital Media"
android:id="@+id/textView"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:background="#2d2291"
android:textColor="#fff155"
android:textAlignment="center"/>
</RelativeLayout>
package uk.co.jonniegrieve.newtestapp;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
public class TestActivity extends AppCompatActivity {
private TextView mText;
@Override
protected void onCreate(Bundle savedInstanceState) {
mText = (TextView) findViewById(R.id.singleText);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_test);
}
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
mText.setText("New Text");
Log.i("Message","Logging click of button");
}
};
}
1 Answer

Seth Kroger
56,413 PointsYou're forgetting to attach the listener to the button. Right now the listener isn't set to any view, so there is no way to trigger it.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsThanks Seth,
It took me a while but with your guidance, I just finished my first Android app (outside the Simple App course).
It took so much trial and error and it worked before I even realise. (My emulator runs very slowly)
I added the code for the button and after a couple of runtime errors itseemed to work after I switched the default ClickListener code so the variables went below it.
I know it's early on in my learning journey but where to do propose I go from here? I'm a little overwhelmed with how much I feel I still have to learn :-)